import requests
import bs4
import pandas as pd
import numpy as np
Lecture 9 - Web Scraping and APIs
Overview
In this lecture, we introduce basic web scraping and API usage to obtain data.
References
This lecture contains material from:
Introduction
Broadly speaking, there are two major paths to reading data from the web
Web scraping: there is data on a webpage, and you want to “scrape” these data
APIs: there is a dedicated server holding data and you want to ask for these data
What is web scraping?
When you type a URL into your browser window, your browser sends a HTTP request to the web server (another computer on the internet whose job it is to respond to requests for the website) to ask for the website’s data. The web server provides the data (e.g. HTML files) which your browser translates into the website you see.
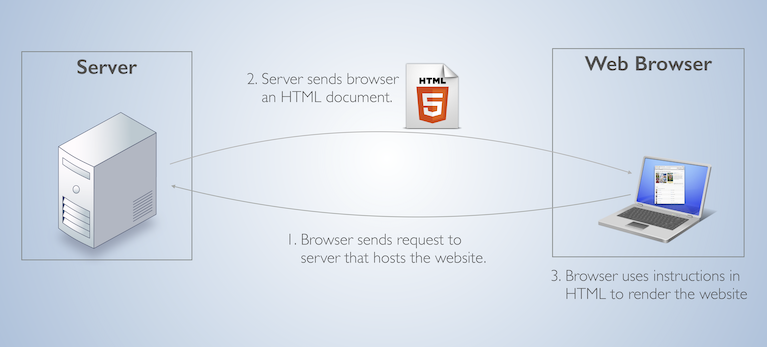
Web scraping is the process of taking information from a website display. Instead of viewing the website data in a browser, we can collect HTML (hypertext markup language) and CSS (cascading style sheet) code and process it to extract the information we want.
- HTML provides the basic structure of a site and tells the browser how to diplay content (eg. titles, tables)
- CSS styles the content and tells the browser how the HTML elements should be presented (e.g. colors, layouts, fonts)
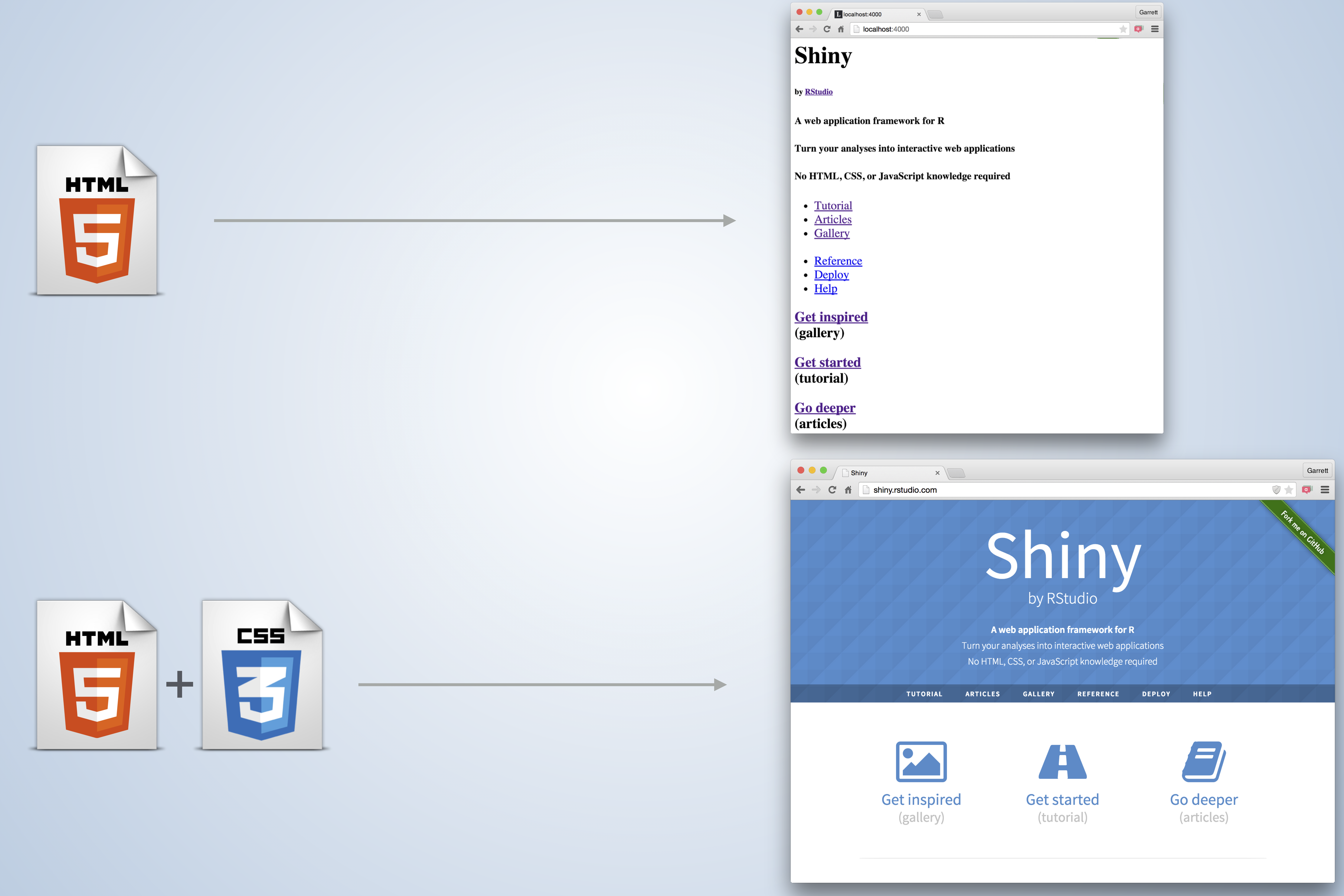
Getting the HTML is generally easy; extracting the data you want from the HTML is usually the difficult part.
For example, below is the source HTML of the Rutgers website.
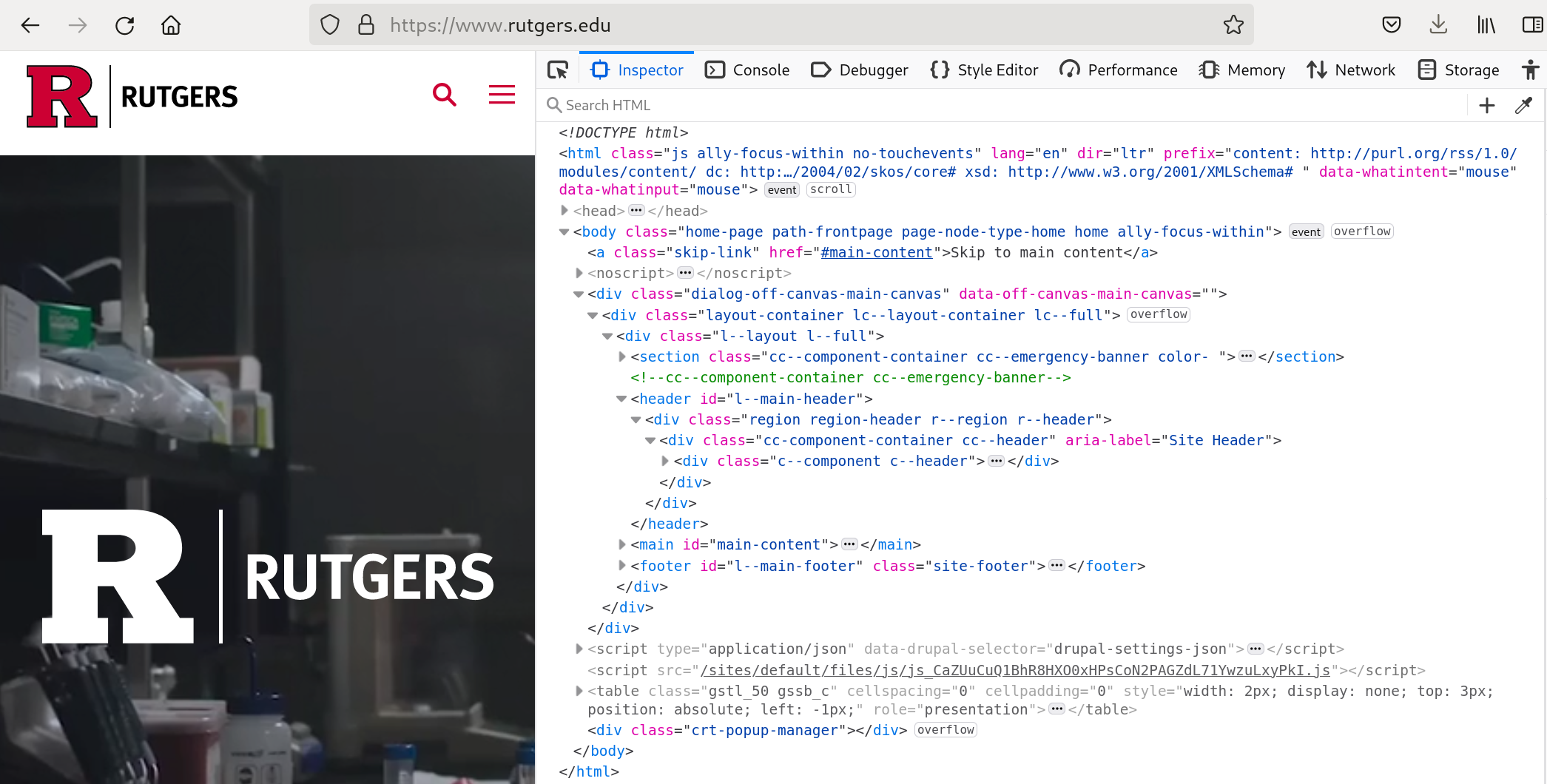
Inspect the https://www.rutgers.edu source code using your web browser.
- Chrome
- View > Developer > View Source
- Safari
- Settings > Advanced > Check “Show features for web developers”
- Develop > Show Page Source
- Microsoft Edge
- Right click > View page source
- Firefox
- Web Developer > Page Source
What are we scraping?
Web scraping is possible because HTML web pages generally have a consistent structure.
Before we scrape any websites, we need to know what HTML files look like. An example:
html
<html>
<head>
<title>Page title</title>
</head>
<body>
<h1 id="first">A heading</h1>
<p class="editor-note">My cat is grumpy</p>
<img src='myimg.png' width='100' height='100'>
</body>
</html>
HTML has a hierarchical structure formed by elements which generally consist of:
- the opening tag: (e.g.
<p>
) the name of the element, wrapped in opening and closing angle brackets - the closing tag: (e.g.
</p>
) the same as the opening tag except with a forward slash before the element name - contents: (e.g.
My cat is grumpy
) the content of the element (everything between start and end tags) - attributes (optional): (e.g.
class="editor-note"
) attributes contain extra information of the element that you don’t want to appear in content. Here.class
is the attribute name andeditor-note
is the attribute value.
Every HTML page must be in an <html>
element and it must have two children <head>
, which contains document metadata like the page title, and <body>
, which contains the content you see in the browser.
Other common elements include:
Block tags like
<h1>
(heading 1),<section>
(section),<p>
(paragraph), and<ol>
(ordered list) form the overall structure of the page.Inline tags like
<b>
(bold),<i>
(italics), and<a>
(link) format text inside block tags.
Some elements have no content and are called void elements. For example, <img>
is a void element.
MDN Web Docs is a good resource for learning more HTML - here is a basic webpage example.
Attributes
In web scraping, attributes are particularly useful.
Two important attributes are id
and class
. These attributes are used in conjunction with CSS to control the visual appearance of the page. For example, any element with class editor-note
may be displayed in a particular way (as specified by CSS). This sort of pattern is known as a CSS selector.
For our purposes, often the data we want to scrape have the same class or CSS selector (because the web page designer wanted these data to look the same!) This is VERY helpful for parsing the HTML soup we often get!
Are we allowed to scrape?
Adapted from R for Data Science (2e), Wickham et al. (2023).
- Is webscraping legal and/or ethical?
Overall, the situation is complicated. Legalities depend on where you live. Generally, if the data is public, non-personal, and factual, you’re likely to be ok.
If the data isn’t public, non-personal, or factual or you’re scraping the data specifically to make money with it, you’ll need to talk to a lawyer.
In any case, you should be respectful; if you are scraping many pages, you should wait a little while between each request. Also, some web servers will block you if you send many requests at once.
- Terms of service
Many websites include a “terms and conditions” or “terms of service” link somewhere on the page, and if you read that page closely you’ll often discover that the site specifically prohibits web scraping.
US: courts have generally found that you are not bound by terms of service (e.g., HiQ Labs v. LinkedIn) if you do not need an account to access the data, and you do not check a box agreeing to terms of service.
Europe: courts have found the terms of service are enforceable even if you do not explicitly agree to them.
- Personally identifiable information
Even if the data is public, you should be extremely careful about scraping personally identifiable information like names, email addresses, phone numbers, dates of birth, etc.
Europe has particularly strict laws about the collection or storage of such data (GDPR).
Regardless of where you live, such scraping is quite likely problematic from an ethical point of view.
In 2016, a group of researchers scraped public profile information (e.g., usernames, age, gender, location, etc.) about 70,000 people on the dating site OkCupid and they publicly released these data without any attempts for anonymization. While the researchers felt that there was nothing wrong with this since the data were already public, this work was widely condemned due to ethics concerns around identifiability of users whose information was released in the dataset. If your work involves scraping personally identifiable information, we strongly recommend reading about the OkCupid study as well as similar studies with questionable research ethics involving the acquisition and release of personally identifiable information.
- Copyright
You also need to worry about copyright law.
US law: copyright protects : “…original works of authorship fixed in any tangible medium of expression…”. It then goes on to describe specific categories that it applies like literary works, musical works, motion pictures and more. Notably absent from copyright protection are data. This means that as long as you limit your scraping to facts, copyright protection does not apply.
As a brief example, in the US, lists of ingredients and instructions are not copyrightable, so copyright can not be used to protect a recipe. But if that list of recipes is accompanied by substantial novel literary content, that is copyrightable.
US fair use: If you do need to scrape original content (like text or images), you may still be protected under the doctrine of fair use. Fair use is not a hard and fast rule, but weighs up a number of factors. It’s more likely to apply if you are collecting the data for research or non-commercial purposes and if you limit what you scrape to just what you need.
European law: Europe has a separate “sui generis” right that protects databases.
How do we scrape?
To get started scraping, you need the URL of the page you want to scrape, which you can usually copy from your web browser.
Then, we can make use of the following packages:
requests
: make a HTTP request to get the website HTMLBeautiful Soup
: parse an HTML file to find data in specific parts of the file
First, install these packages in your conda environment:
pip install requests
pip install beautifulsoup4
Example: Rutgers Statistics Faculty
The requests.get
function downloads the HTML source code.
= "https://statistics.rutgers.edu/people-pages/faculty"
url = requests.get(url)
response response
<Response [200]>
NOTE: Response [200] indicates successful response.
The raw data (as bytes) is stored in response.content
:
# b' ' indicates bytes
response.content
b'<!DOCTYPE html>\n<html lang="en-gb" dir="ltr">\n<head>\n\t<meta charset="utf-8">\n\t<meta name="viewport" content="width=device-width, initial-scale=1">\n\t<meta name="description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey">\n\t<meta name="generator" content="Joomla! - Open Source Content Management">\n\t<title>Faculty</title>\n\t<link href="/media/templates/site/cassiopeia_sas/images/favicon.ico" rel="alternate icon" type="image/vnd.microsoft.icon">\n\t<link href="/media/system/images/joomla-favicon-pinned.svg" rel="mask-icon" color="#000">\n\n\t<link href="/media/system/css/joomla-fontawesome.min.css?62ec5d" rel="lazy-stylesheet"><noscript><link href="/media/system/css/joomla-fontawesome.min.css?62ec5d" rel="stylesheet"></noscript>\n\t<link href="/media/cache/com_latestnewsenhancedpro/style_articles_blog_100570.css?62ec5d" rel="stylesheet">\n\t<link href="/media/syw/css/fonts.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/vendor/chosen/css/chosen.css?1.8.7" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/template.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/global/colors_standard.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/vendor/joomla-custom-elements/joomla-alert.min.css?0.2.0" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia_sas/css/user.css?62ec5d" rel="stylesheet">\n\t<link href="/media/plg_system_jcepro/site/css/content.min.css?86aa0286b6232c4a5b58f892ce080277" rel="stylesheet">\n\t<style>@media (min-width: 768px) {#lnepmodal .modal-dialog {width: 80%;max-width: 600px;}}</style>\n\t<style>:root {\n\t\t--hue: 214;\n\t\t--template-bg-light: #f0f4fb;\n\t\t--template-text-dark: #495057;\n\t\t--template-text-light: #ffffff;\n\t\t--template-link-color: #2a69b8;\n\t\t--template-special-color: #001B4C;\n\t\t\n\t}</style>\n\t<style>\n#accordeonck276 { padding:0;margin:0;padding-left: 0px;-moz-border-radius: 0px 0px 0px 0px;-webkit-border-radius: 0px 0px 0px 0px;border-radius: 0px 0px 0px 0px;-moz-box-shadow: 0px 0px 0px 0px #444444;-webkit-box-shadow: 0px 0px 0px 0px #444444;box-shadow: 0px 0px 0px 0px #444444;border-top: none;border-right: none;border-bottom: none;border-left: none; } \n#accordeonck276 li.accordeonck { list-style: none;overflow: hidden; }\n#accordeonck276 ul[class^="content"] { margin:0;padding:0; }\n#accordeonck276 li.accordeonck > span { position: relative; display: block; }\n#accordeonck276 li.accordeonck.parent > span { padding-right: 15px;}\n#accordeonck276 li.parent > span span.toggler_icon { position: absolute; cursor: pointer; display: block; height: 100%; z-index: 10;right:0; background: url(/media/templates/site/cassiopeia_sas/images/arrow-down.png) center center no-repeat !important;width: 15px;}\n#accordeonck276 li.parent.open > span span.toggler_icon { right:0; background: url(/media/templates/site/cassiopeia_sas/images/arrow-up.png) center center no-repeat !important;}\n#accordeonck276 li.accordeonck.level2 > span { padding-right: 20px;}\n#accordeonck276 li.level2 li.accordeonck > span { padding-right: 20px;}\n#accordeonck276 a.accordeonck { display: block;text-decoration: none; color: #000000;font-size: 17px;}\n#accordeonck276 a.accordeonck:hover { text-decoration: none; color: #CC0033;}\n#accordeonck276 li.parent > span a { display: block;outline: none; }\n#accordeonck276 li.parent.open > span a { }\n#accordeonck276 a.accordeonck > .badge { margin: 0 0 0 5px; }\n#accordeonck276 li.level2.parent.open > span span.toggler_icon { background: url(/) center center no-repeat !important;}\n#accordeonck276 li.level3.parent.open > span span.toggler_icon { background: url(/) center center no-repeat !important;}\n#accordeonck276 li.level1 { padding-top: 5px;padding-bottom: 5px; } \n#accordeonck276 li.level1 > span { border-bottom: #DDDDDD 1px solid ; } \n#accordeonck276 li.level1 > span a { padding-top: 5px;padding-right: 5px;padding-bottom: 5px;padding-left: 5px;color: #000000;font-size: 17px; } \n#accordeonck276 li.level1 > span span.accordeonckdesc { font-size: 10px; } \n#accordeonck276 li.level1:hover > span { border-left: #CC0033 5px solid ; } \n#accordeonck276 li.level1:hover > span a { color: #CC0033; } \n#accordeonck276 li.level1.active > span { border-left: #CC0033 5px solid ; } \n#accordeonck276 li.level1.active > span a { color: #CC0033; } \n#accordeonck276 li.level1.active > span { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level1.active > span a { } \n#accordeonck276 li.level1 > ul { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 > span { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 > span a { padding-top: 5px;padding-bottom: 5px;padding-left: 15px;color: #000000;font-size: 16px; } \n#accordeonck276 li.level2:hover > span { } \n#accordeonck276 li.level2:hover > span a { color: #000000; } \n#accordeonck276 li.level2.active > span { } \n#accordeonck276 li.level2.active > span a { color: #000000; } \n#accordeonck276 li.level2 ul[class^="content"] { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 li.accordeonck > span { } \n#accordeonck276 li.level2 li.accordeonck > span a { padding-top: 5px;padding-bottom: 5px;padding-left: 25px;color: #636363; } \n#accordeonck276 li.level2 li.accordeonck:hover > span { } \n#accordeonck276 li.level2 li.accordeonck:hover > span a { color: #000000; } \n#accordeonck276 li.level2 li.accordeonck.active > span { } \n#accordeonck276 li.level2 li.accordeonck.active > span a { color: #000000; } ul#accordeonck276 li.accordeonck.level1.active {\nbackground:#ddd; }\n\na.accordeonck.isactive {\n font-weight: bold;\n}\n\n.accordeonck li.parent > span span.toggler_icon {\n z-index: 9 !important;\n}\naccordeonck276 li.accordeonck.level1.active {\nbackground:#ddd; }\n\na.accordeonck.isactive {\n font-weight: bold;\n}\n\n.accordeonck li.parent > span span.toggler_icon {\n z-index: 9 !important;\n}\n#accordeonck276 ul[class^="content"] {\r\n\tdisplay: none;\r\n}\r\n\r\n#accordeonck276 .toggler_icon {\r\n\ttop: 0;;\r\n}</style>\n\n\t<script src="/media/vendor/metismenujs/js/metismenujs.min.js?1.4.0" defer></script>\n\t<script type="application/json" class="joomla-script-options new">{"bootstrap.tooltip":{".hasTooltip":{"animation":true,"container":"body","html":true,"trigger":"hover focus","boundary":"clippingParents","sanitize":true}},"joomla.jtext":{"RLTA_BUTTON_SCROLL_LEFT":"Scroll buttons to the left","RLTA_BUTTON_SCROLL_RIGHT":"Scroll buttons to the right","ERROR":"Error","MESSAGE":"Message","NOTICE":"Notice","WARNING":"Warning","JCLOSE":"Close","JOK":"OK","JOPEN":"Open"},"system.paths":{"root":"","rootFull":"https://statistics.rutgers.edu/","base":"","baseFull":"https://statistics.rutgers.edu/"},"csrf.token":"adc719b3fae21b13fbbddd9df4795d1b"}</script>\n\t<script src="/media/system/js/core.min.js?2cb912"></script>\n\t<script src="/media/vendor/webcomponentsjs/js/webcomponents-bundle.min.js?2.8.0" nomodule defer></script>\n\t<script src="/media/vendor/bootstrap/js/popover.min.js?5.3.3" type="module"></script>\n\t<script src="/media/vendor/bootstrap/js/modal.min.js?5.3.3" type="module"></script>\n\t<script src="/media/templates/site/cassiopeia/js/template.min.js?62ec5d" type="module"></script>\n\t<script src="/media/vendor/jquery/js/jquery.min.js?3.7.1"></script>\n\t<script src="/media/vendor/chosen/js/chosen.jquery.min.js?1.8.7"></script>\n\t<script src="/media/legacy/js/joomla-chosen.min.js?62ec5d"></script>\n\t<script src="/media/legacy/js/jquery-noconflict.min.js?504da4"></script>\n\t<script src="/media/vendor/bootstrap/js/offcanvas.min.js?5.3.3" type="module"></script>\n\t<script src="/media/templates/site/cassiopeia/js/mod_menu/menu-metismenu.min.js?62ec5d" defer></script>\n\t<script src="/media/system/js/joomla-hidden-mail.min.js?80d9c7" type="module"></script>\n\t<script src="/media/system/js/messages.min.js?9a4811" type="module"></script>\n\t<script src="/media/com_accordeonmenuck/assets/accordeonmenuck.js"></script>\n\t<script src="/media/com_accordeonmenuck/assets/jquery.easing.1.3.js"></script>\n\t<script>\n\t\tjQuery(document).ready(function (){\n\t\t\tjQuery(\'select\').jchosen({"disable_search_threshold":10,"search_contains":true,"allow_single_deselect":true,"placeholder_text_multiple":"Type or select some options","placeholder_text_single":"Select an option","no_results_text":"No results match"});\n\t\t});\n\t</script>\n\t<script>function clearForm() { document.getElementById(\'filter-search\').value=\'\'; document.adminForm.limitstart.value=0; document.adminForm.submit(); } </script>\n\t<script>document.addEventListener("readystatechange", function(event) {if (event.target.readyState === "complete") {var modal = document.getElementById("lnepmodal");modal.addEventListener("show.bs.modal", function (event) {var link = event.relatedTarget;if (typeof (link) !== "undefined" && link !== null) {var dataTitle = link.getAttribute("data-modaltitle");if (typeof (dataTitle) !== "undefined" && dataTitle !== null) {this.querySelector(".modal-title").innerText = dataTitle;}var dataURL = link.getAttribute("href");this.querySelector(".iframe").setAttribute("src", dataURL);}document.querySelector("body").classList.add("modal-open");var event = document.createEvent("Event"); event.initEvent("modalopen", true, true); document.dispatchEvent(event);}, this);modal.addEventListener("shown.bs.modal", function (event) {var modal_body = this.querySelector(".modal-body");}, this);modal.addEventListener("hide.bs.modal", function (event) {this.querySelector(".modal-title").innerText = "Article";var modal_body = this.querySelector(".modal-body");modal_body.querySelector(".iframe").setAttribute("src", "about:blank");document.querySelector("body").classList.remove("modal-open");var event = document.createEvent("Event"); event.initEvent("modalclose", true, true); document.dispatchEvent(event);}, this);}});</script>\n\t<script>rltaSettings = {"switchToAccordions":true,"switchBreakPoint":576,"buttonScrollSpeed":5,"addHashToUrls":true,"rememberActive":false,"wrapButtons":false}</script>\n\t<script>jQuery(document).ready(function(jQuery){new Accordeonmenuck(\'#accordeonck276\', {fadetransition : false,eventtype : \'click\',transition : \'linear\',menuID : \'accordeonck276\',defaultopenedid : \'\',activeeffect : \'\',showcounter : \'\',showactive : \'1\',closeothers : \'1\',duree : 500});}); </script>\n\t<meta property="og:locale" content="en_GB" class="4SEO_ogp_tag">\n\t<meta property="og:url" content="https://statistics.rutgers.edu/people-pages/faculty" class="4SEO_ogp_tag">\n\t<meta property="og:site_name" content="Rutgers University :: Department of Statistics and Biostatistics" class="4SEO_ogp_tag">\n\t<meta property="og:type" content="article" class="4SEO_ogp_tag">\n\t<meta property="og:title" content="Faculty" class="4SEO_ogp_tag">\n\t<meta property="og:description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey" class="4SEO_ogp_tag">\n\t<meta property="fb:app_id" content="966242223397117" class="4SEO_ogp_tag">\n\t<meta property="og:image" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_ogp_tag">\n\t<meta property="og:image:width" content="300" class="4SEO_ogp_tag">\n\t<meta property="og:image:height" content="300" class="4SEO_ogp_tag">\n\t<meta property="og:image:alt" content="Pierre Bellec" class="4SEO_ogp_tag">\n\t<meta property="og:image:secure_url" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_ogp_tag">\n\t<meta name="twitter:card" content="summary" class="4SEO_tcards_tag">\n\t<meta name="twitter:url" content="https://statistics.rutgers.edu/people-pages/faculty" class="4SEO_tcards_tag">\n\t<meta name="twitter:title" content="Faculty" class="4SEO_tcards_tag">\n\t<meta name="twitter:description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey" class="4SEO_tcards_tag">\n\t<meta name="twitter:image" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_tcards_tag">\n\n<!-- Global site tag (gtag.js) - Google Analytics -->\n<script async src="https://www.googletagmanager.com/gtag/js?id=G-V859C9HTED"></script>\n\n<script>\nwindow.dataLayer = window.dataLayer || [];function gtag(){dataLayer.push(arguments);}gtag(\'js\', new Date()); gtag(\'config\', \'G-V859C9HTED\');\n</script>\n\t<meta name="robots" content="max-snippet:-1, max-image-preview:large, max-video-preview:-1" class="4SEO_robots_tag">\n\t<script type="application/ld+json" class="4SEO_structured_data_breadcrumb">{\n "@context": "http://schema.org",\n "@type": "BreadcrumbList",\n "itemListElement": [\n {\n "@type": "listItem",\n "position": 1,\n "name": "Home",\n "item": "https://statistics.rutgers.edu/"\n },\n {\n "@type": "listItem",\n "position": 2,\n "name": "Faculty",\n "item": "https://statistics.rutgers.edu/people-pages/faculty"\n }\n ]\n}</script></head>\n\n<body class="site com_latestnewsenhancedpro wrapper-static view-articles no-layout no-task itemid-100570 has-sidebar-left">\n\t<header class="header container-header full-width">\n\n\t\t \n \n \t\t\t<div class="container-sas-branding ">\n\t\t\t\t<div class="sas-branding no-card ">\n \n<div id="mod-custom259" class="mod-custom custom">\n <div class="container-logo">\r\n<div class="row">\r\n<div><a href="https://sas.rutgers.edu" target="_blank" rel="noopener"><img alt="RU Logo 2024" class="theme-image" /></a></div>\r\n</div>\r\n</div>\r\n<div class="container-unit">\r\n<div class="row">\r\n<div class="col title-unit"><a href="/." class="no-underline no-hover">Department of Statistics</a></div>\r\n</div>\r\n</div></div>\n</div>\n<div class="sas-branding no-card d-none d-xl-block">\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar-sas-ru">\n<li class="metismenu-item item-101205 level-1"><a href="https://sas.rutgers.edu/about/events/upcoming-events" target="_blank" rel="noopener noreferrer">SAS Events</a></li><li class="metismenu-item item-101206 level-1"><a href="https://sas.rutgers.edu/about/news" target="_blank" rel="noopener noreferrer">SAS News</a></li><li class="metismenu-item item-100002 level-1"><a href="https://www.rutgers.edu" target="_blank" rel="noopener noreferrer">rutgers.edu</a></li><li class="metismenu-item item-100118 level-1"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener noreferrer">SAS</a></li><li class="metismenu-item item-100009 level-1"><a href="https://search.rutgers.edu/people" target="_blank" rel="noopener noreferrer">Search People</a></li><li class="metismenu-item item-100010 level-1"><a href="/search-website" ><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="" width="25" height="24" loading="lazy"><span class="image-title visually-hidden">Search Website</span></a></li></ul>\n</div>\n\n\t\t\t</div>\n\t\t \n\t\t\t\t\t<div class="grid-child">\n\t\t\t\t<div class="navbar-brand">\n\t\t\t\t\t<a class="brand-logo" href="/">\n\t\t\t\t\t\t<img class="logo d-inline-block" loading="eager" decoding="async" src="/media/templates/site/cassiopeia/images/logo.svg" alt="Rutgers University :: Department of Statistics and Biostatistics">\t\t\t\t\t</a>\n\t\t\t\t\t\t\t\t\t</div>\n\t\t\t</div>\n\t\t \n \n\t\t\n\t\t\t\t\t<div class="container-banner full-width">\n\t\t\t\t<div class="banner card menu-bar">\n <div class="card-body">\n <nav class="navbar navbar-expand-lg">\n <button class="navbar-toggler navbar-toggler-right" type="button" data-bs-toggle="offcanvas" data-bs-target="#navbar256" aria-controls="navbar256" aria-expanded="false" aria-label="Toggle Navigation">\n <span class="icon-menu" aria-hidden="true"></span>\n </button>\n <div class="offcanvas offcanvas-start" id="navbar256">\n <div class="offcanvas-header">\n <button type="button" class="btn-close btn-close-black" data-bs-dismiss="offcanvas" aria-label="Close"></button>\n\n \n </div>\n <div class="offcanvas-body">\n <div class="d-lg-none mt-3">\n <div class="moduletable ">\n \n<div id="mod-custom260" class="mod-custom custom">\n <p style="text-align: center;"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener"><img alt="RU Logo 2024" class="theme-image" style="max-width:80%" /></a><br /><a href="/." class="no-underline no-hover title-unit">Department of Statistics</a></p>\r\n<p><a href="/./search-website"><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="Search" style="display: block; margin-left: auto; margin-right: auto;" /></a></p></div>\n</div>\n </div>\n\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar navbar-nav dropdown sas-main-menu">\n<li class="metismenu-item item-100829 level-1"><a href="/welcome" >Welcome</a></li><li class="metismenu-item item-100136 level-1 divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">Academics</button><ul class="mm-collapse"><li class="metismenu-item item-100138 level-2"><a href="/why-statistics" >Undergraduate Statistics</a></li><li class="metismenu-item item-100918 level-2"><a href="https://mps.rutgers.edu/data-science" target="_blank" rel="noopener noreferrer">Undergraduate Data Science</a></li><li class="metismenu-item item-100925 level-2"><a href="/academics/ms-statistics" >MS Statistics</a></li><li class="metismenu-item item-100924 level-2"><a href="https://msds-stat.rutgers.edu/" target="_blank" rel="noopener noreferrer">Professional MSDS</a></li><li class="metismenu-item item-100923 level-2"><a href="https://www.fsrm.rutgers.edu/" target="_blank" rel="noopener noreferrer">Professional FSRM</a></li><li class="metismenu-item item-100137 level-2"><a href="/academics/phd-statistics" >PhD Statistics</a></li></ul></li><li class="metismenu-item item-100277 level-1 active divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">People</button><ul class="mm-collapse"><li class="metismenu-item item-101303 level-2"><a href="/people-pages/administrative-team" >Administrative Team</a></li><li class="metismenu-item item-100570 level-2 current active"><a href="/people-pages/faculty" aria-current="page">Faculty</a></li><li class="metismenu-item item-100919 level-2"><a href="/people-pages/open-rank-faculty-positions" >Two Open Rank Faculty Positions</a></li><li class="metismenu-item item-101304 level-2"><a href="/people-pages/teaching-faculty-and-lecturers" >Teaching Faculty and Lecturers</a></li><li class="metismenu-item item-100575 level-2"><a href="/people-pages/affiliated-faculty" >Affiliated Faculty</a></li><li class="metismenu-item item-101305 level-2"><a href="/people-pages/graduate-students" >Ph.D. Students and Post Docs</a></li><li class="metismenu-item item-101306 level-2"><a href="/people-pages/recent-graduates" >Recent PhD Graduates</a></li><li class="metismenu-item item-100573 level-2"><a href="/people-pages/staff" >Staff</a></li><li class="metismenu-item item-100572 level-2"><a href="/people-pages/emeritus" >Emeritus</a></li></ul></li><li class="metismenu-item item-100342 level-1 divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">News & Events</button><ul class="mm-collapse"><li class="metismenu-item item-111 level-2"><a href="/news-events/news" >News</a></li><li class="metismenu-item item-166 level-2"><a href="/news-events/seminars" >Seminars</a></li><li class="metismenu-item item-175 level-2"><a href="/news-events/conferences" >Conferences</a></li></ul></li><li class="metismenu-item item-100577 level-1 deeper parent"><a href="/osc-home" >Consulting</a><button class="mm-collapsed mm-toggler mm-toggler-link" aria-haspopup="true" aria-expanded="false" aria-label="Consulting"></button><ul class="mm-collapse"><li class="metismenu-item item-100591 level-2"><a href="/osc-home" >Office of Statistical Consulting</a></li></ul></li><li class="metismenu-item item-100650 level-1"><a href="https://give.rutgersfoundation.org/applied-statistics/1015.html" target="_blank" rel="noopener noreferrer">Support Us</a></li><li class="metismenu-item item-100341 level-1"><a href="/contact-us" >Contact Us</a></li></ul>\n\n\n </div>\n </div>\n</nav> </div>\n</div>\n\n\t\t\t</div>\n\t\t\n\n\t</header>\n \n \n \n\t<div class="site-grid">\n\n\t\t\n\t\t\n\t\t\n\t\t\t\t\t<div class="grid-child container-sidebar-left">\n\t\t\t\t<div class="sidebar-left card ">\n <h3 class="card-header ">People</h3> <div class="card-body">\n <div class="accordeonck-wrap" data-id="accordeonck276">\n<ul class="menu" id="accordeonck276">\n<li id="item-101303" class="accordeonck item101303 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/administrative-team" >Administrative Team<span class="accordeonckdesc"></span></a></span></li><li id="item-100570" class="accordeonck item100570 current active level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck isactive " href="/people-pages/faculty" >Faculty<span class="accordeonckdesc"></span></a></span></li><li id="item-100919" class="accordeonck item100919 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/open-rank-faculty-positions" >Two Open Rank Faculty Positions<span class="accordeonckdesc"></span></a></span></li><li id="item-101304" class="accordeonck item101304 level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/teaching-faculty-and-lecturers" >Teaching Faculty and Lecturers<span class="accordeonckdesc"></span></a></span></li><li id="item-100575" class="accordeonck item100575 level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/affiliated-faculty" >Affiliated Faculty<span class="accordeonckdesc"></span></a></span></li><li id="item-101305" class="accordeonck item101305 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/graduate-students" >Ph.D. Students and Post Docs<span class="accordeonckdesc"></span></a></span></li><li id="item-101306" class="accordeonck item101306 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/recent-graduates" >Recent PhD Graduates<span class="accordeonckdesc"></span></a></span></li><li id="item-100573" class="accordeonck item100573 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/staff" >Staff<span class="accordeonckdesc"></span></a></span></li><li id="item-100572" class="accordeonck item100572 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/emeritus" >Emeritus<span class="accordeonckdesc"></span></a></span></li></ul></div>\n </div>\n</div>\n\n\t\t\t</div>\n\t\t\n\t\t<div class="grid-child container-component">\n\t\t\t\n\t\t\t\n\t\t\t<div id="system-message-container" aria-live="polite"></div>\n\n\t\t\t<main>\n\t\t\t\t<div class="lnep_blog">\r\n\t\t\t<div class="page-header">\r\n\t\t\t<h1>Faculty</h1>\r\n\t\t</div>\r\n\t\r\n\t\r\n\t\r\n\t<form action="/people-pages/faculty" method="get" name="adminForm" id="adminForm">\r\n\r\n\t\t<input type="hidden" name="option" value="com_latestnewsenhancedpro">\r\n\t\t<input type="hidden" name="view" value="articles">\r\n\t\t<input type="hidden" name="category" value="" />\r\n\t\t<input type="hidden" name="tag" value="" />\r\n\t\t<input type="hidden" name="author" value="" />\r\n\t\t<input type="hidden" name="alias" value="" />\r\n\t\t<input type="hidden" name="period" value="" />\r\n\t\t<input type="hidden" name="stage" value="" />\r\n\t\t\t\t<input type="hidden" name="limitstart" value="0" />\r\n\t\t<input type="hidden" name="filter_order" value="" />\r\n\t\t<input type="hidden" name="filter_order_Dir" value="ASC" />\r\n\t\t<input type="hidden" name="adc719b3fae21b13fbbddd9df4795d1b" value="1">\r\n\t\t\t\t\t<div class="pagination_wrapper top">\r\n\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t<div class="searchblock">\r\n \t \t \t \t <div class="row mb-3">\r\n \t<div class="col-auto">\r\n\t \t<div class="input-group me-1">\r\n\t \t\t<label for="filter-search" class="visually-hidden">Search</label>\r\n\t \t\t<input type="text" name="filter-search" id="filter-search" class="form-control" placeholder="Enter text here" value="" />\r\n\t \t\t<button type="button" aria-label="Search" class="btn btn-primary hasTooltip" name="searchbtn" id="searchbtn" onclick="document.adminForm.limitstart.value=0;this.form.submit(); return false;" title="Search"><i class="SYWicon-search"></i></button>\r\n\t \t</div>\r\n\t </div>\r\n \t<div class="col-auto">\r\n \t\t\t\t<button type="button" class="btn btn-primary" onclick="clearForm(); return false;">Clear</button>\r\n\t </div>\r\n </div>\r\n\t\t\t \t </div>\r\n <script>\r\n\t\tdocument.getElementById("filter-search").addEventListener("keyup", function(event) { event.preventDefault(); if (event.keyCode === 13) { document.getElementById("searchbtn").click(); } });\r\n </script>\r\n\t\t\t\t\r\n\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t</div>\r\n\t\t\r\n\t\t\t\t\t<div class="latestnews-items">\r\n\t\t\t\t <div class="latestnews-item id-368 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/368-pierre-bellec" class="hasTooltip" title="Pierre Bellec" aria-label="Read more about Pierre Bellec"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Pierre Bellec" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/368-pierre-bellec" class="hasTooltip" title="Pierre Bellec" aria-label="Read more about Pierre Bellec"> <span>Pierre Bellec</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:pcb71@stat.rutgers.edu">pcb71@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-715 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/715-matteo-bonvini" class="hasTooltip" title="Matteo Bonvini" aria-label="Read more about Matteo Bonvini"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_715.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Matteo Bonvini" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/715-matteo-bonvini" class="hasTooltip" title="Matteo Bonvini" aria-label="Read more about Matteo Bonvini"> <span>Matteo Bonvini</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mb1662@stat.rutgers.edu">mb1662@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-369 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/369-steven-buyske" class="hasTooltip" title="Steve Buyske" aria-label="Read more about Steve Buyske"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_369.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Steve Buyske" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/369-steven-buyske" class="hasTooltip" title="Steve Buyske" aria-label="Read more about Steve Buyske"> <span>Steve Buyske</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor; Co-Director of Undergraduate Statistics Program; Co-Director of Undergraduate Data Science Program</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:buyske@stat.rutgers.edu">buyske@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-370 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/370-javier-cabrera" class="hasTooltip" title="Javier Cabrera" aria-label="Read more about Javier Cabrera"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_370.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Javier Cabrera" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/370-javier-cabrera" class="hasTooltip" title="Javier Cabrera" aria-label="Read more about Javier Cabrera"> <span>Javier Cabrera</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:cabrera@stat.rutgers.edu">cabrera@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-371 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/371-rong-chen" class="hasTooltip" title="Rong Chen" aria-label="Read more about Rong Chen"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_371.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Rong Chen" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/371-rong-chen" class="hasTooltip" title="Rong Chen" aria-label="Read more about Rong Chen"> <span>Rong Chen</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Chair</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:rongchen@stat.rutgers.edu">rongchen@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-650 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/650-yaqing-chen" class="hasTooltip" title="Yaqing Chen" aria-label="Read more about Yaqing Chen"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_650.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Yaqing Chen" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/650-yaqing-chen" class="hasTooltip" title="Yaqing Chen" aria-label="Read more about Yaqing Chen"> <span>Yaqing Chen</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:yqchen@stat.rutgers.edu">yqchen@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-373 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/373-harry-crane" class="hasTooltip" title="Harry Crane" aria-label="Read more about Harry Crane"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_373.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Harry Crane" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/373-harry-crane" class="hasTooltip" title="Harry Crane" aria-label="Read more about Harry Crane"> <span>Harry Crane</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:hcrane@stat.rutgers.edu">hcrane@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-374 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/374-tirthankar-dasgupta" class="hasTooltip" title="Tirthankar DasGupta" aria-label="Read more about Tirthankar DasGupta"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_374.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Tirthankar DasGupta" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/374-tirthankar-dasgupta" class="hasTooltip" title="Tirthankar DasGupta" aria-label="Read more about Tirthankar DasGupta"> <span>Tirthankar DasGupta</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Graduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:tirthankar.dasgupta@rutgers.edu">tirthankar.dasgupta@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-403 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/403-ruobin-gong" class="hasTooltip" title="Ruobin Gong" aria-label="Read more about Ruobin Gong"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_403.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Ruobin Gong" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/403-ruobin-gong" class="hasTooltip" title="Ruobin Gong" aria-label="Read more about Ruobin Gong"> <span>Ruobin Gong</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ruobin.gong@rutgers.edu">ruobin.gong@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-400 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/400-zijian-guo" class="hasTooltip" title="Zijian Guo" aria-label="Read more about Zijian Guo"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_400.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Zijian Guo" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/400-zijian-guo" class="hasTooltip" title="Zijian Guo" aria-label="Read more about Zijian Guo"> <span>Zijian Guo</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:zijguo@stat.rutgers.edu">zijguo@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-399 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/399-qiyang-han" class="hasTooltip" title="Qiyang Han" aria-label="Read more about Qiyang Han"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_399.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Qiyang Han" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/399-qiyang-han" class="hasTooltip" title="Qiyang Han" aria-label="Read more about Qiyang Han"> <span>Qiyang Han</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:qh85@stat.rutgers.edu">qh85@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-398 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/398-donald-r-hoover" class="hasTooltip" title="Donald R. Hoover" aria-label="Read more about Donald R. Hoover"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_398.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Donald R. Hoover" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/398-donald-r-hoover" class="hasTooltip" title="Donald R. Hoover" aria-label="Read more about Donald R. Hoover"> <span>Donald R. Hoover</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:drhoover@stat.rutgers.edu">drhoover@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-397 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/397-ying-hung" class="hasTooltip" title="Ying Hung" aria-label="Read more about Ying Hung"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_397.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Ying Hung" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/397-ying-hung" class="hasTooltip" title="Ying Hung" aria-label="Read more about Ying Hung"> <span>Ying Hung</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:yhung@stat.rutgers.edu">yhung@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-651 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/651-koulik-khamaru" class="hasTooltip" title="Koulik Khamaru" aria-label="Read more about Koulik Khamaru"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_651.jpeg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Koulik Khamaru" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/651-koulik-khamaru" class="hasTooltip" title="Koulik Khamaru" aria-label="Read more about Koulik Khamaru"> <span>Koulik Khamaru</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:kk1241@stat.rutgers.edu">kk1241@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-395 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/395-john-kolassa" class="hasTooltip" title="John Kolassa" aria-label="Read more about John Kolassa"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_395.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="John Kolassa" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/395-john-kolassa" class="hasTooltip" title="John Kolassa" aria-label="Read more about John Kolassa"> <span>John Kolassa</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:kolassa@stat.rutgers.edu">kolassa@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-391 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/391-regina-y-liu" class="hasTooltip" title="Regina Y. Liu" aria-label="Read more about Regina Y. Liu"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_391.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Regina Y. Liu" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/391-regina-y-liu" class="hasTooltip" title="Regina Y. Liu" aria-label="Read more about Regina Y. Liu"> <span>Regina Y. Liu</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:rliu@stat.rutgers.edu">rliu@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-716 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/716-gemma-moran" class="hasTooltip" title="Gemma Moran" aria-label="Read more about Gemma Moran"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_716.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Gemma Moran" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/716-gemma-moran" class="hasTooltip" title="Gemma Moran" aria-label="Read more about Gemma Moran"> <span>Gemma Moran</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:gm845@stat.rutgers.edu">gm845@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-573 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/573-nicole-pashley" class="hasTooltip" title="Nicole Pashley" aria-label="Read more about Nicole Pashley"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_573.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Nicole Pashley" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/573-nicole-pashley" class="hasTooltip" title="Nicole Pashley" aria-label="Read more about Nicole Pashley"> <span>Nicole Pashley</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:np755@stat.rutgers.edu">np755@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-387 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/387-harold-b-sackrowitz" class="hasTooltip" title="Harold B. Sackrowitz" aria-label="Read more about Harold B. Sackrowitz"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_387.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Harold B. Sackrowitz" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/387-harold-b-sackrowitz" class="hasTooltip" title="Harold B. Sackrowitz" aria-label="Read more about Harold B. Sackrowitz"> <span>Harold B. Sackrowitz</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Undergraduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:sackrowi@stat.rutgers.edu">sackrowi@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-542 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/542-michael-l-stein" class="hasTooltip" title="Michael L. Stein" aria-label="Read more about Michael L. Stein"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_542.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Michael L. Stein" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/542-michael-l-stein" class="hasTooltip" title="Michael L. Stein" aria-label="Read more about Michael L. Stein"> <span>Michael L. Stein</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ms2870@stat.rutgers.edu">ms2870@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-383 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/383-zhiqiang-tan" class="hasTooltip" title="Zhiqiang Tan" aria-label="Read more about Zhiqiang Tan"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_383.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Zhiqiang Tan" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/383-zhiqiang-tan" class="hasTooltip" title="Zhiqiang Tan" aria-label="Read more about Zhiqiang Tan"> <span>Zhiqiang Tan</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor </span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ztan@stat.rutgers.edu">ztan@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-382 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/382-david-e-tyler" class="hasTooltip" title="David E. Tyler" aria-label="Read more about David E. Tyler"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_382.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="David E. Tyler" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/382-david-e-tyler" class="hasTooltip" title="David E. Tyler" aria-label="Read more about David E. Tyler"> <span>David E. Tyler</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:dtyler@stat.rutgers.edu">dtyler@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-572 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/572-guanyang-wang" class="hasTooltip" title="Guanyang Wang" aria-label="Read more about Guanyang Wang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_572.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Guanyang Wang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/572-guanyang-wang" class="hasTooltip" title="Guanyang Wang" aria-label="Read more about Guanyang Wang"> <span>Guanyang Wang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:guanyang.wang@rutgers.edu">guanyang.wang@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-381 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/381-sijan-wang" class="hasTooltip" title="Sijian Wang" aria-label="Read more about Sijian Wang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_381.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Sijian Wang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/381-sijan-wang" class="hasTooltip" title="Sijian Wang" aria-label="Read more about Sijian Wang"> <span>Sijian Wang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Director of FSRM and MSDS programs</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:sijian.wang@stat.rutgers.edu">sijian.wang@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-380 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/380-han-xiao" class="hasTooltip" title="Han Xiao" aria-label="Read more about Han Xiao"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_380.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Han Xiao" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/380-han-xiao" class="hasTooltip" title="Han Xiao" aria-label="Read more about Han Xiao"> <span>Han Xiao</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Graduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:hxiao@stat.rutgers.edu">hxiao@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-379 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/379-minge-xie" class="hasTooltip" title="Minge Xie" aria-label="Read more about Minge Xie"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_379.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Minge Xie" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/379-minge-xie" class="hasTooltip" title="Minge Xie" aria-label="Read more about Minge Xie"> <span>Minge Xie</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Director, Office of Statistical Consulting</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mxie@stat.rutgers.edu">mxie@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-378 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/378-min-xu" class="hasTooltip" title="Min Xu" aria-label="Read more about Min Xu"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_378.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Min Xu" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/378-min-xu" class="hasTooltip" title="Min Xu" aria-label="Read more about Min Xu"> <span>Min Xu</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mx76@stat.rutgers.edu">mx76@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-376 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/376-cun-hui-zhang" class="hasTooltip" title="Cun-Hui Zhang" aria-label="Read more about Cun-Hui Zhang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_376.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Cun-Hui Zhang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/376-cun-hui-zhang" class="hasTooltip" title="Cun-Hui Zhang" aria-label="Read more about Cun-Hui Zhang"> <span>Cun-Hui Zhang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Co-Director of FSRM and MSDS programs</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:czhang@stat.rutgers.edu">czhang@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-546 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/546-linjun-zhang" class="hasTooltip" title="Linjun Zhang" aria-label="Read more about Linjun Zhang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_546.jpeg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Linjun Zhang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/546-linjun-zhang" class="hasTooltip" title="Linjun Zhang" aria-label="Read more about Linjun Zhang"> <span>Linjun Zhang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:linjun.zhang@rutgers.edu">linjun.zhang@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> \t\t\t</div>\r\n\t\t\r\n\t\t<div class="pagination_wrapper bottom">\r\n\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t<div class="counterpagination">\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t</div>\r\n\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t</div>\r\n\t</form>\r\n\r\n\t</div>\r\n\t<div id="lnepmodal" data-backdrop="false" data-keyboard="true" data-remote="true" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="lnepmodalLabel" aria-hidden="true">\r\n \t<div class="modal-dialog" role="document">\r\n \t\t<div class="modal-content">\r\n \t<div class="modal-header">\r\n \t\t \t\t \t\t \t\t\t<h5 id="lnepmodalLabel" class="modal-title">Article</h5>\r\n \t\t\t<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>\r\n \t\t \t</div>\r\n \t<div class="modal-body">\r\n \t\t<iframe class="iframe" height="500" style="display: block; width: 100%; border: 0; max-height: none; overflow: auto"></iframe>\r\n \t</div>\r\n \t<div class="modal-footer">\r\n \t\t<button class="btn btn-secondary" data-bs-dismiss="modal" aria-hidden="true">Close</button>\r\n \t</div>\r\n \t\t</div>\r\n \t</div>\r\n </div>\n\t\t\t</main>\n \t\t \t\t \t\t\t\t</div>\n\n\t\t\n\t\t\n\t\t \n \n \t\t \n \t\t\t</div>\n\n\t \n \n\t\n\t \n \n \t\t\t<sas-footer-identity class=" grid-child container-sas-footer-identity full-width-v2">\n\t\t\t<div class=" sas-footer-identity">\n\t\t\t\t<div class="sas-footer-identity no-card sas-footer-logo-left sas-footer-logo-left sas-branding">\n \n<div id="mod-custom261" class="mod-custom custom">\n <p><img src="/media/templates/site/cassiopeia_sas/images/RNBSAS_H_WHITE.svg" alt="White RU Logo" style="min-width: 300px; max-width: 400px; margin-top: 10px; margin-left: 15px; margin-bottom: 10px;" loading="lazy" /></p></div>\n</div>\n<div class="sas-footer-identity no-card ">\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list mod-menu mod-list nav navbar-sas-ru sas-footer-menu sas-footer-menu-right">\n<li class="metismenu-item item-101205 level-1"><a href="https://sas.rutgers.edu/about/events/upcoming-events" target="_blank" rel="noopener noreferrer">SAS Events</a></li><li class="metismenu-item item-101206 level-1"><a href="https://sas.rutgers.edu/about/news" target="_blank" rel="noopener noreferrer">SAS News</a></li><li class="metismenu-item item-100002 level-1"><a href="https://www.rutgers.edu" target="_blank" rel="noopener noreferrer">rutgers.edu</a></li><li class="metismenu-item item-100118 level-1"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener noreferrer">SAS</a></li><li class="metismenu-item item-100009 level-1"><a href="https://search.rutgers.edu/people" target="_blank" rel="noopener noreferrer">Search People</a></li><li class="metismenu-item item-100010 level-1"><a href="/search-website" ><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="" width="25" height="24" loading="lazy"><span class="image-title visually-hidden">Search Website</span></a></li></ul>\n</div>\n\n\t\t\t</div>\n\t\t</sas-footer-identity>\n\t\n<footer class="container-footer footer full-width">\n \n \n <!-- Display any module in the footer position from the sites --> \n<div class="grid-child">\n<!--2025-01 LG: Display Rutgers Menu from rutgers file --> \n<div><h3 class="title">Connect with Rutgers</h3>\n<ul class="list-unstyled">\n<li><a href="https://newbrunswick.rutgers.edu/" target="_blank" >Rutgers New Brunswick</a>\n<li><a href="https://www.rutgers.edu/news" target="blank">Rutgers Today</a>\n<li><a href="https://my.rutgers.edu/uxp/login" target="_blank">myRutgers</a>\n<li><a href="https://scheduling.rutgers.edu/scheduling/academic-calendar" target="_blank">Academic Calendar</a>\n<li><a href="https://classes.rutgers.edu//soc/#home" target="_blank">Rutgers Schedule of Classes</a>\n<li><a href="https://emnb.rutgers.edu/one-stop-overview/" target="_blank">One Stop Student Service Center</a>\n<li><a href="https://rutgers.campuslabs.com/engage/events/" target="_blank">getINVOLVED</a>\n<li><a href="https://admissions.rutgers.edu/visit-rutgers" target="_blank">Plan a Visit</a>\n</ul>\n</div> \n <!--2025-01 LG: Display SAS Menu from SAS file --> \n <div>\n <h3 class="title">Explore SAS</h3>\n<ul class="list-unstyled">\n<li><a href="https://sas.rutgers.edu/academics/majors-minors" target="blank">Majors and Minors</a>\n<li><a href="https://sas.rutgers.edu/academics/areas-of-study" target="_blank" >Departments and Programs</a>\n<li><a href="https://sas.rutgers.edu/academics/centers-institutes" target="_blank">Research Centers and Institutes</a>\n<li><a href="https://sas.rutgers.edu/about/sas-offices" target="_blank">SAS Offices</a> \n<li><a href="https://sas.rutgers.edu/giving" target="_blank">Support SAS</a>\n</ul>\n</div> \n \n<!--2025-01 LG: Display NOTICES Menu from NOTICES file --> \n<div>\n<h3 class="title">Notices</h3>\n<ul class="list-unstyled">\n<li><a href="https://www.rutgers.edu/status" target="_blank" >University Operating Status</a>\n</ul>\n<hr>\n<ul class="list-unstyled">\n<li><a href="https://www.rutgers.edu/privacy-statement" target="blank">Privacy</a>\n</ul>\n</div> \n <div class="moduletable ">\n <h3 class="title">Contact Us</h3> \n<div id="mod-custom96" class="mod-custom custom">\n <p><img src="/images/stories/hill2_70x70.jpg" alt="hill2_70x70" width="90" height="90" style="margin-right: 10px; margin-bottom: 5px; margin-top: 10px; float: left;" />501 Hill Center<br />110 Frelinghuysen Road<br />Piscataway, NJ 08854<br /><strong>_____________________________</strong><br />Phone: 848-445-2690</p>\r\n<p>General Inquiries: <joomla-hidden-mail is-link="1" is-email="1" first="YWRtaW4=" last="c3RhdC5ydXRnZXJzLmVkdQ==" text="YWRtaW5Ac3RhdC5ydXRnZXJzLmVkdQ==" base="" >This email address is being protected from spambots. You need JavaScript enabled to view it.</joomla-hidden-mail><br />Special Permission: <a href="https://secure.sas.rutgers.edu/apps/special_permission/" target="_blank" rel="noopener">https://secure.sas.rutgers.edu/apps/special_permission/</a><br /><joomla-hidden-mail is-link="1" is-email="1" first="ZXNoYXJrZXk=" last="c3RhdC5ydXRnZXJzLmVkdQ==" text="ZXNoYXJrZXlAc3RhdC5ydXRnZXJzLmVkdQ==" base="" >This email address is being protected from spambots. You need JavaScript enabled to view it.</joomla-hidden-mail></p></div>\n</div>\n\n\t</div>\n</footer>\n\n<!-- Display Social Media Module --> \n\t \n\n<!--Display Footer Menu --> \n \n\t\t\t<sas-footer-menu class="container-sas-footer-menu full-width">\n\t\t\t<div class="sas-footer-menu">\n\t\t\t\t<ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar navbar-nav dropdown sas-footer-menu">\n<li class="metismenu-item item-100003 level-1"><a href="/" >Home</a></li><li class="metismenu-item item-100052 level-1"><a href="https://ithelp.sas.rutgers.edu/" target="_blank" rel="noopener noreferrer">IT Help</a></li><li class="metismenu-item item-100119 level-1"><a href="/contact-us" >Contact Us</a></li><li class="metismenu-item item-100639 level-1"><a href="/site-map?view=html&id=2" >Site Map</a></li><li class="metismenu-item item-100827 level-1"><a href="/search-in-footer" >Search</a></li><li class="metismenu-item item-100772 level-1"><a href="https://statistics.rutgers.edu/?morequest=sso&idp=urn:mace:incommon:rutgers.edu" >Login</a></li></ul>\n\n\t\t\t</div>\n\t\t</sas-footer-menu>\n\t\n\n<!-- Display Copyright -->\n\t\t<copyright class="container-sas-copyright full-width">\n\t\t\t<div class="sas-copyright">\n\n<!--2025-01 LG: Display Copyright Text from copyright text file --> \n<p>\n <!-- paragraph 1 of copy right - information -->\n<p style="text-align: center;">Rutgers is an equal access/equal opportunity institution. Individuals with disabilities are encouraged to direct suggestions, comments, or complaints concerning any<br />\naccessibility issues with Rutgers websites to <a href=\'mailto:accessibility@rutgers.edu\'>accessibility@rutgers.edu</a> or complete the <a href=\'https://it.rutgers.edu/it-accessibility-initiative/barrierform/\' rel=\'nofollow\' target=\'_blank\'>Report Accessibility Barrier / Provide Feedback</a> form.</p>\n <!-- paragraph 2 of copy right - information -->\n<p style="text-align: center;"><a href=\'https://www.rutgers.edu/copyright-information\' rel=\'nofollow\' target=\'_blank\'>Copyright \xc2\xa9<script>document.write(new Date().getFullYear())</script></a>, <a href=\'https://www.rutgers.edu/\' rel=\'nofollow\' target=\'_blank\'>Rutgers, The State University of New Jersey</a>. All rights reserved. <a href=\'https://ithelp.sas.rutgers.edu/\' rel=\'nofollow\' target=\'_blank\'>Contact webmaster</a></p>\n<p> </p>\n\n</p>\n\n<!-- Display back to top link --> \n\t\t\t<a href="#top" id="back-top" class="back-to-top-link" aria-label="Back to Top">\n\t\t\t<span class="icon-arrow-up icon-fw" aria-hidden="true"></span>\n\t\t</a>\n\n\t\n\n<noscript class="4SEO_cron">\n <img aria-hidden="true" alt="" style="position:absolute;bottom:0;left:0;z-index:-99999;" src="https://statistics.rutgers.edu/index.php/_wblapi?nolangfilter=1&_wblapi=/forseo/v1/cron/image/" data-pagespeed-no-transform data-speed-no-transform />\n</noscript>\n<script class="4SEO_cron" data-speed-no-transform >setTimeout(function () {\n var e = document.createElement(\'img\');\n e.setAttribute(\'style\', \'position:absolute;bottom:0;right:0;z-index:-99999\');\n e.setAttribute(\'aria-hidden\', \'true\');\n e.setAttribute(\'src\', \'https://statistics.rutgers.edu/index.php/_wblapi?nolangfilter=1&_wblapi=/forseo/v1/cron/image/\' + Math.random().toString().substring(2) + Math.random().toString().substring(2) + \'.svg\');\n document.body.appendChild(e);\n setTimeout(function () {\n document.body.removeChild(e)\n }, 3000)\n }, 3000);\n</script>\n</body>\n</html>\n'
response.text
decodes the binary content and returns a string:
response.text
'<!DOCTYPE html>\n<html lang="en-gb" dir="ltr">\n<head>\n\t<meta charset="utf-8">\n\t<meta name="viewport" content="width=device-width, initial-scale=1">\n\t<meta name="description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey">\n\t<meta name="generator" content="Joomla! - Open Source Content Management">\n\t<title>Faculty</title>\n\t<link href="/media/templates/site/cassiopeia_sas/images/favicon.ico" rel="alternate icon" type="image/vnd.microsoft.icon">\n\t<link href="/media/system/images/joomla-favicon-pinned.svg" rel="mask-icon" color="#000">\n\n\t<link href="/media/system/css/joomla-fontawesome.min.css?62ec5d" rel="lazy-stylesheet"><noscript><link href="/media/system/css/joomla-fontawesome.min.css?62ec5d" rel="stylesheet"></noscript>\n\t<link href="/media/cache/com_latestnewsenhancedpro/style_articles_blog_100570.css?62ec5d" rel="stylesheet">\n\t<link href="/media/syw/css/fonts.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/vendor/chosen/css/chosen.css?1.8.7" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/template.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/global/colors_standard.min.css?62ec5d" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia/css/vendor/joomla-custom-elements/joomla-alert.min.css?0.2.0" rel="stylesheet">\n\t<link href="/media/templates/site/cassiopeia_sas/css/user.css?62ec5d" rel="stylesheet">\n\t<link href="/media/plg_system_jcepro/site/css/content.min.css?86aa0286b6232c4a5b58f892ce080277" rel="stylesheet">\n\t<style>@media (min-width: 768px) {#lnepmodal .modal-dialog {width: 80%;max-width: 600px;}}</style>\n\t<style>:root {\n\t\t--hue: 214;\n\t\t--template-bg-light: #f0f4fb;\n\t\t--template-text-dark: #495057;\n\t\t--template-text-light: #ffffff;\n\t\t--template-link-color: #2a69b8;\n\t\t--template-special-color: #001B4C;\n\t\t\n\t}</style>\n\t<style>\n#accordeonck276 { padding:0;margin:0;padding-left: 0px;-moz-border-radius: 0px 0px 0px 0px;-webkit-border-radius: 0px 0px 0px 0px;border-radius: 0px 0px 0px 0px;-moz-box-shadow: 0px 0px 0px 0px #444444;-webkit-box-shadow: 0px 0px 0px 0px #444444;box-shadow: 0px 0px 0px 0px #444444;border-top: none;border-right: none;border-bottom: none;border-left: none; } \n#accordeonck276 li.accordeonck { list-style: none;overflow: hidden; }\n#accordeonck276 ul[class^="content"] { margin:0;padding:0; }\n#accordeonck276 li.accordeonck > span { position: relative; display: block; }\n#accordeonck276 li.accordeonck.parent > span { padding-right: 15px;}\n#accordeonck276 li.parent > span span.toggler_icon { position: absolute; cursor: pointer; display: block; height: 100%; z-index: 10;right:0; background: url(/media/templates/site/cassiopeia_sas/images/arrow-down.png) center center no-repeat !important;width: 15px;}\n#accordeonck276 li.parent.open > span span.toggler_icon { right:0; background: url(/media/templates/site/cassiopeia_sas/images/arrow-up.png) center center no-repeat !important;}\n#accordeonck276 li.accordeonck.level2 > span { padding-right: 20px;}\n#accordeonck276 li.level2 li.accordeonck > span { padding-right: 20px;}\n#accordeonck276 a.accordeonck { display: block;text-decoration: none; color: #000000;font-size: 17px;}\n#accordeonck276 a.accordeonck:hover { text-decoration: none; color: #CC0033;}\n#accordeonck276 li.parent > span a { display: block;outline: none; }\n#accordeonck276 li.parent.open > span a { }\n#accordeonck276 a.accordeonck > .badge { margin: 0 0 0 5px; }\n#accordeonck276 li.level2.parent.open > span span.toggler_icon { background: url(/) center center no-repeat !important;}\n#accordeonck276 li.level3.parent.open > span span.toggler_icon { background: url(/) center center no-repeat !important;}\n#accordeonck276 li.level1 { padding-top: 5px;padding-bottom: 5px; } \n#accordeonck276 li.level1 > span { border-bottom: #DDDDDD 1px solid ; } \n#accordeonck276 li.level1 > span a { padding-top: 5px;padding-right: 5px;padding-bottom: 5px;padding-left: 5px;color: #000000;font-size: 17px; } \n#accordeonck276 li.level1 > span span.accordeonckdesc { font-size: 10px; } \n#accordeonck276 li.level1:hover > span { border-left: #CC0033 5px solid ; } \n#accordeonck276 li.level1:hover > span a { color: #CC0033; } \n#accordeonck276 li.level1.active > span { border-left: #CC0033 5px solid ; } \n#accordeonck276 li.level1.active > span a { color: #CC0033; } \n#accordeonck276 li.level1.active > span { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level1.active > span a { } \n#accordeonck276 li.level1 > ul { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 > span { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 > span a { padding-top: 5px;padding-bottom: 5px;padding-left: 15px;color: #000000;font-size: 16px; } \n#accordeonck276 li.level2:hover > span { } \n#accordeonck276 li.level2:hover > span a { color: #000000; } \n#accordeonck276 li.level2.active > span { } \n#accordeonck276 li.level2.active > span a { color: #000000; } \n#accordeonck276 li.level2 ul[class^="content"] { background: #DDDDDD;background-color: #DDDDDD;background: -moz-linear-gradient(top, #DDDDDD 0%, #DDDDDD 100%);background: -webkit-gradient(linear, left top, left bottom, color-stop(0%,#DDDDDD), color-stop(100%,#DDDDDD)); background: -webkit-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -o-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: -ms-linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%);background: linear-gradient(top, #DDDDDD 0%,#DDDDDD 100%); } \n#accordeonck276 li.level2 li.accordeonck > span { } \n#accordeonck276 li.level2 li.accordeonck > span a { padding-top: 5px;padding-bottom: 5px;padding-left: 25px;color: #636363; } \n#accordeonck276 li.level2 li.accordeonck:hover > span { } \n#accordeonck276 li.level2 li.accordeonck:hover > span a { color: #000000; } \n#accordeonck276 li.level2 li.accordeonck.active > span { } \n#accordeonck276 li.level2 li.accordeonck.active > span a { color: #000000; } ul#accordeonck276 li.accordeonck.level1.active {\nbackground:#ddd; }\n\na.accordeonck.isactive {\n font-weight: bold;\n}\n\n.accordeonck li.parent > span span.toggler_icon {\n z-index: 9 !important;\n}\naccordeonck276 li.accordeonck.level1.active {\nbackground:#ddd; }\n\na.accordeonck.isactive {\n font-weight: bold;\n}\n\n.accordeonck li.parent > span span.toggler_icon {\n z-index: 9 !important;\n}\n#accordeonck276 ul[class^="content"] {\r\n\tdisplay: none;\r\n}\r\n\r\n#accordeonck276 .toggler_icon {\r\n\ttop: 0;;\r\n}</style>\n\n\t<script src="/media/vendor/metismenujs/js/metismenujs.min.js?1.4.0" defer></script>\n\t<script type="application/json" class="joomla-script-options new">{"bootstrap.tooltip":{".hasTooltip":{"animation":true,"container":"body","html":true,"trigger":"hover focus","boundary":"clippingParents","sanitize":true}},"joomla.jtext":{"RLTA_BUTTON_SCROLL_LEFT":"Scroll buttons to the left","RLTA_BUTTON_SCROLL_RIGHT":"Scroll buttons to the right","ERROR":"Error","MESSAGE":"Message","NOTICE":"Notice","WARNING":"Warning","JCLOSE":"Close","JOK":"OK","JOPEN":"Open"},"system.paths":{"root":"","rootFull":"https://statistics.rutgers.edu/","base":"","baseFull":"https://statistics.rutgers.edu/"},"csrf.token":"adc719b3fae21b13fbbddd9df4795d1b"}</script>\n\t<script src="/media/system/js/core.min.js?2cb912"></script>\n\t<script src="/media/vendor/webcomponentsjs/js/webcomponents-bundle.min.js?2.8.0" nomodule defer></script>\n\t<script src="/media/vendor/bootstrap/js/popover.min.js?5.3.3" type="module"></script>\n\t<script src="/media/vendor/bootstrap/js/modal.min.js?5.3.3" type="module"></script>\n\t<script src="/media/templates/site/cassiopeia/js/template.min.js?62ec5d" type="module"></script>\n\t<script src="/media/vendor/jquery/js/jquery.min.js?3.7.1"></script>\n\t<script src="/media/vendor/chosen/js/chosen.jquery.min.js?1.8.7"></script>\n\t<script src="/media/legacy/js/joomla-chosen.min.js?62ec5d"></script>\n\t<script src="/media/legacy/js/jquery-noconflict.min.js?504da4"></script>\n\t<script src="/media/vendor/bootstrap/js/offcanvas.min.js?5.3.3" type="module"></script>\n\t<script src="/media/templates/site/cassiopeia/js/mod_menu/menu-metismenu.min.js?62ec5d" defer></script>\n\t<script src="/media/system/js/joomla-hidden-mail.min.js?80d9c7" type="module"></script>\n\t<script src="/media/system/js/messages.min.js?9a4811" type="module"></script>\n\t<script src="/media/com_accordeonmenuck/assets/accordeonmenuck.js"></script>\n\t<script src="/media/com_accordeonmenuck/assets/jquery.easing.1.3.js"></script>\n\t<script>\n\t\tjQuery(document).ready(function (){\n\t\t\tjQuery(\'select\').jchosen({"disable_search_threshold":10,"search_contains":true,"allow_single_deselect":true,"placeholder_text_multiple":"Type or select some options","placeholder_text_single":"Select an option","no_results_text":"No results match"});\n\t\t});\n\t</script>\n\t<script>function clearForm() { document.getElementById(\'filter-search\').value=\'\'; document.adminForm.limitstart.value=0; document.adminForm.submit(); } </script>\n\t<script>document.addEventListener("readystatechange", function(event) {if (event.target.readyState === "complete") {var modal = document.getElementById("lnepmodal");modal.addEventListener("show.bs.modal", function (event) {var link = event.relatedTarget;if (typeof (link) !== "undefined" && link !== null) {var dataTitle = link.getAttribute("data-modaltitle");if (typeof (dataTitle) !== "undefined" && dataTitle !== null) {this.querySelector(".modal-title").innerText = dataTitle;}var dataURL = link.getAttribute("href");this.querySelector(".iframe").setAttribute("src", dataURL);}document.querySelector("body").classList.add("modal-open");var event = document.createEvent("Event"); event.initEvent("modalopen", true, true); document.dispatchEvent(event);}, this);modal.addEventListener("shown.bs.modal", function (event) {var modal_body = this.querySelector(".modal-body");}, this);modal.addEventListener("hide.bs.modal", function (event) {this.querySelector(".modal-title").innerText = "Article";var modal_body = this.querySelector(".modal-body");modal_body.querySelector(".iframe").setAttribute("src", "about:blank");document.querySelector("body").classList.remove("modal-open");var event = document.createEvent("Event"); event.initEvent("modalclose", true, true); document.dispatchEvent(event);}, this);}});</script>\n\t<script>rltaSettings = {"switchToAccordions":true,"switchBreakPoint":576,"buttonScrollSpeed":5,"addHashToUrls":true,"rememberActive":false,"wrapButtons":false}</script>\n\t<script>jQuery(document).ready(function(jQuery){new Accordeonmenuck(\'#accordeonck276\', {fadetransition : false,eventtype : \'click\',transition : \'linear\',menuID : \'accordeonck276\',defaultopenedid : \'\',activeeffect : \'\',showcounter : \'\',showactive : \'1\',closeothers : \'1\',duree : 500});}); </script>\n\t<meta property="og:locale" content="en_GB" class="4SEO_ogp_tag">\n\t<meta property="og:url" content="https://statistics.rutgers.edu/people-pages/faculty" class="4SEO_ogp_tag">\n\t<meta property="og:site_name" content="Rutgers University :: Department of Statistics and Biostatistics" class="4SEO_ogp_tag">\n\t<meta property="og:type" content="article" class="4SEO_ogp_tag">\n\t<meta property="og:title" content="Faculty" class="4SEO_ogp_tag">\n\t<meta property="og:description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey" class="4SEO_ogp_tag">\n\t<meta property="fb:app_id" content="966242223397117" class="4SEO_ogp_tag">\n\t<meta property="og:image" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_ogp_tag">\n\t<meta property="og:image:width" content="300" class="4SEO_ogp_tag">\n\t<meta property="og:image:height" content="300" class="4SEO_ogp_tag">\n\t<meta property="og:image:alt" content="Pierre Bellec" class="4SEO_ogp_tag">\n\t<meta property="og:image:secure_url" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_ogp_tag">\n\t<meta name="twitter:card" content="summary" class="4SEO_tcards_tag">\n\t<meta name="twitter:url" content="https://statistics.rutgers.edu/people-pages/faculty" class="4SEO_tcards_tag">\n\t<meta name="twitter:title" content="Faculty" class="4SEO_tcards_tag">\n\t<meta name="twitter:description" content="The School of Arts and Sciences, Rutgers, The State University of New Jersey" class="4SEO_tcards_tag">\n\t<meta name="twitter:image" content="https://statistics.rutgers.edu/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" class="4SEO_tcards_tag">\n\n<!-- Global site tag (gtag.js) - Google Analytics -->\n<script async src="https://www.googletagmanager.com/gtag/js?id=G-V859C9HTED"></script>\n\n<script>\nwindow.dataLayer = window.dataLayer || [];function gtag(){dataLayer.push(arguments);}gtag(\'js\', new Date()); gtag(\'config\', \'G-V859C9HTED\');\n</script>\n\t<meta name="robots" content="max-snippet:-1, max-image-preview:large, max-video-preview:-1" class="4SEO_robots_tag">\n\t<script type="application/ld+json" class="4SEO_structured_data_breadcrumb">{\n "@context": "http://schema.org",\n "@type": "BreadcrumbList",\n "itemListElement": [\n {\n "@type": "listItem",\n "position": 1,\n "name": "Home",\n "item": "https://statistics.rutgers.edu/"\n },\n {\n "@type": "listItem",\n "position": 2,\n "name": "Faculty",\n "item": "https://statistics.rutgers.edu/people-pages/faculty"\n }\n ]\n}</script></head>\n\n<body class="site com_latestnewsenhancedpro wrapper-static view-articles no-layout no-task itemid-100570 has-sidebar-left">\n\t<header class="header container-header full-width">\n\n\t\t \n \n \t\t\t<div class="container-sas-branding ">\n\t\t\t\t<div class="sas-branding no-card ">\n \n<div id="mod-custom259" class="mod-custom custom">\n <div class="container-logo">\r\n<div class="row">\r\n<div><a href="https://sas.rutgers.edu" target="_blank" rel="noopener"><img alt="RU Logo 2024" class="theme-image" /></a></div>\r\n</div>\r\n</div>\r\n<div class="container-unit">\r\n<div class="row">\r\n<div class="col title-unit"><a href="/." class="no-underline no-hover">Department of Statistics</a></div>\r\n</div>\r\n</div></div>\n</div>\n<div class="sas-branding no-card d-none d-xl-block">\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar-sas-ru">\n<li class="metismenu-item item-101205 level-1"><a href="https://sas.rutgers.edu/about/events/upcoming-events" target="_blank" rel="noopener noreferrer">SAS Events</a></li><li class="metismenu-item item-101206 level-1"><a href="https://sas.rutgers.edu/about/news" target="_blank" rel="noopener noreferrer">SAS News</a></li><li class="metismenu-item item-100002 level-1"><a href="https://www.rutgers.edu" target="_blank" rel="noopener noreferrer">rutgers.edu</a></li><li class="metismenu-item item-100118 level-1"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener noreferrer">SAS</a></li><li class="metismenu-item item-100009 level-1"><a href="https://search.rutgers.edu/people" target="_blank" rel="noopener noreferrer">Search People</a></li><li class="metismenu-item item-100010 level-1"><a href="/search-website" ><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="" width="25" height="24" loading="lazy"><span class="image-title visually-hidden">Search Website</span></a></li></ul>\n</div>\n\n\t\t\t</div>\n\t\t \n\t\t\t\t\t<div class="grid-child">\n\t\t\t\t<div class="navbar-brand">\n\t\t\t\t\t<a class="brand-logo" href="/">\n\t\t\t\t\t\t<img class="logo d-inline-block" loading="eager" decoding="async" src="/media/templates/site/cassiopeia/images/logo.svg" alt="Rutgers University :: Department of Statistics and Biostatistics">\t\t\t\t\t</a>\n\t\t\t\t\t\t\t\t\t</div>\n\t\t\t</div>\n\t\t \n \n\t\t\n\t\t\t\t\t<div class="container-banner full-width">\n\t\t\t\t<div class="banner card menu-bar">\n <div class="card-body">\n <nav class="navbar navbar-expand-lg">\n <button class="navbar-toggler navbar-toggler-right" type="button" data-bs-toggle="offcanvas" data-bs-target="#navbar256" aria-controls="navbar256" aria-expanded="false" aria-label="Toggle Navigation">\n <span class="icon-menu" aria-hidden="true"></span>\n </button>\n <div class="offcanvas offcanvas-start" id="navbar256">\n <div class="offcanvas-header">\n <button type="button" class="btn-close btn-close-black" data-bs-dismiss="offcanvas" aria-label="Close"></button>\n\n \n </div>\n <div class="offcanvas-body">\n <div class="d-lg-none mt-3">\n <div class="moduletable ">\n \n<div id="mod-custom260" class="mod-custom custom">\n <p style="text-align: center;"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener"><img alt="RU Logo 2024" class="theme-image" style="max-width:80%" /></a><br /><a href="/." class="no-underline no-hover title-unit">Department of Statistics</a></p>\r\n<p><a href="/./search-website"><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="Search" style="display: block; margin-left: auto; margin-right: auto;" /></a></p></div>\n</div>\n </div>\n\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar navbar-nav dropdown sas-main-menu">\n<li class="metismenu-item item-100829 level-1"><a href="/welcome" >Welcome</a></li><li class="metismenu-item item-100136 level-1 divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">Academics</button><ul class="mm-collapse"><li class="metismenu-item item-100138 level-2"><a href="/why-statistics" >Undergraduate Statistics</a></li><li class="metismenu-item item-100918 level-2"><a href="https://mps.rutgers.edu/data-science" target="_blank" rel="noopener noreferrer">Undergraduate Data Science</a></li><li class="metismenu-item item-100925 level-2"><a href="/academics/ms-statistics" >MS Statistics</a></li><li class="metismenu-item item-100924 level-2"><a href="https://msds-stat.rutgers.edu/" target="_blank" rel="noopener noreferrer">Professional MSDS</a></li><li class="metismenu-item item-100923 level-2"><a href="https://www.fsrm.rutgers.edu/" target="_blank" rel="noopener noreferrer">Professional FSRM</a></li><li class="metismenu-item item-100137 level-2"><a href="/academics/phd-statistics" >PhD Statistics</a></li></ul></li><li class="metismenu-item item-100277 level-1 active divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">People</button><ul class="mm-collapse"><li class="metismenu-item item-101303 level-2"><a href="/people-pages/administrative-team" >Administrative Team</a></li><li class="metismenu-item item-100570 level-2 current active"><a href="/people-pages/faculty" aria-current="page">Faculty</a></li><li class="metismenu-item item-100919 level-2"><a href="/people-pages/open-rank-faculty-positions" >Two Open Rank Faculty Positions</a></li><li class="metismenu-item item-101304 level-2"><a href="/people-pages/teaching-faculty-and-lecturers" >Teaching Faculty and Lecturers</a></li><li class="metismenu-item item-100575 level-2"><a href="/people-pages/affiliated-faculty" >Affiliated Faculty</a></li><li class="metismenu-item item-101305 level-2"><a href="/people-pages/graduate-students" >Ph.D. Students and Post Docs</a></li><li class="metismenu-item item-101306 level-2"><a href="/people-pages/recent-graduates" >Recent PhD Graduates</a></li><li class="metismenu-item item-100573 level-2"><a href="/people-pages/staff" >Staff</a></li><li class="metismenu-item item-100572 level-2"><a href="/people-pages/emeritus" >Emeritus</a></li></ul></li><li class="metismenu-item item-100342 level-1 divider deeper parent"><button class="mod-menu__separator separator mm-collapsed mm-toggler mm-toggler-nolink" aria-haspopup="true" aria-expanded="false">News & Events</button><ul class="mm-collapse"><li class="metismenu-item item-111 level-2"><a href="/news-events/news" >News</a></li><li class="metismenu-item item-166 level-2"><a href="/news-events/seminars" >Seminars</a></li><li class="metismenu-item item-175 level-2"><a href="/news-events/conferences" >Conferences</a></li></ul></li><li class="metismenu-item item-100577 level-1 deeper parent"><a href="/osc-home" >Consulting</a><button class="mm-collapsed mm-toggler mm-toggler-link" aria-haspopup="true" aria-expanded="false" aria-label="Consulting"></button><ul class="mm-collapse"><li class="metismenu-item item-100591 level-2"><a href="/osc-home" >Office of Statistical Consulting</a></li></ul></li><li class="metismenu-item item-100650 level-1"><a href="https://give.rutgersfoundation.org/applied-statistics/1015.html" target="_blank" rel="noopener noreferrer">Support Us</a></li><li class="metismenu-item item-100341 level-1"><a href="/contact-us" >Contact Us</a></li></ul>\n\n\n </div>\n </div>\n</nav> </div>\n</div>\n\n\t\t\t</div>\n\t\t\n\n\t</header>\n \n \n \n\t<div class="site-grid">\n\n\t\t\n\t\t\n\t\t\n\t\t\t\t\t<div class="grid-child container-sidebar-left">\n\t\t\t\t<div class="sidebar-left card ">\n <h3 class="card-header ">People</h3> <div class="card-body">\n <div class="accordeonck-wrap" data-id="accordeonck276">\n<ul class="menu" id="accordeonck276">\n<li id="item-101303" class="accordeonck item101303 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/administrative-team" >Administrative Team<span class="accordeonckdesc"></span></a></span></li><li id="item-100570" class="accordeonck item100570 current active level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck isactive " href="/people-pages/faculty" >Faculty<span class="accordeonckdesc"></span></a></span></li><li id="item-100919" class="accordeonck item100919 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/open-rank-faculty-positions" >Two Open Rank Faculty Positions<span class="accordeonckdesc"></span></a></span></li><li id="item-101304" class="accordeonck item101304 level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/teaching-faculty-and-lecturers" >Teaching Faculty and Lecturers<span class="accordeonckdesc"></span></a></span></li><li id="item-100575" class="accordeonck item100575 level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/affiliated-faculty" >Affiliated Faculty<span class="accordeonckdesc"></span></a></span></li><li id="item-101305" class="accordeonck item101305 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/graduate-students" >Ph.D. Students and Post Docs<span class="accordeonckdesc"></span></a></span></li><li id="item-101306" class="accordeonck item101306 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/recent-graduates" >Recent PhD Graduates<span class="accordeonckdesc"></span></a></span></li><li id="item-100573" class="accordeonck item100573 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/staff" >Staff<span class="accordeonckdesc"></span></a></span></li><li id="item-100572" class="accordeonck item100572 first level1 " data-level="1" ><span class="accordeonck_outer "><a class="accordeonck " href="/people-pages/emeritus" >Emeritus<span class="accordeonckdesc"></span></a></span></li></ul></div>\n </div>\n</div>\n\n\t\t\t</div>\n\t\t\n\t\t<div class="grid-child container-component">\n\t\t\t\n\t\t\t\n\t\t\t<div id="system-message-container" aria-live="polite"></div>\n\n\t\t\t<main>\n\t\t\t\t<div class="lnep_blog">\r\n\t\t\t<div class="page-header">\r\n\t\t\t<h1>Faculty</h1>\r\n\t\t</div>\r\n\t\r\n\t\r\n\t\r\n\t<form action="/people-pages/faculty" method="get" name="adminForm" id="adminForm">\r\n\r\n\t\t<input type="hidden" name="option" value="com_latestnewsenhancedpro">\r\n\t\t<input type="hidden" name="view" value="articles">\r\n\t\t<input type="hidden" name="category" value="" />\r\n\t\t<input type="hidden" name="tag" value="" />\r\n\t\t<input type="hidden" name="author" value="" />\r\n\t\t<input type="hidden" name="alias" value="" />\r\n\t\t<input type="hidden" name="period" value="" />\r\n\t\t<input type="hidden" name="stage" value="" />\r\n\t\t\t\t<input type="hidden" name="limitstart" value="0" />\r\n\t\t<input type="hidden" name="filter_order" value="" />\r\n\t\t<input type="hidden" name="filter_order_Dir" value="ASC" />\r\n\t\t<input type="hidden" name="adc719b3fae21b13fbbddd9df4795d1b" value="1">\r\n\t\t\t\t\t<div class="pagination_wrapper top">\r\n\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t<div class="searchblock">\r\n \t \t \t \t <div class="row mb-3">\r\n \t<div class="col-auto">\r\n\t \t<div class="input-group me-1">\r\n\t \t\t<label for="filter-search" class="visually-hidden">Search</label>\r\n\t \t\t<input type="text" name="filter-search" id="filter-search" class="form-control" placeholder="Enter text here" value="" />\r\n\t \t\t<button type="button" aria-label="Search" class="btn btn-primary hasTooltip" name="searchbtn" id="searchbtn" onclick="document.adminForm.limitstart.value=0;this.form.submit(); return false;" title="Search"><i class="SYWicon-search"></i></button>\r\n\t \t</div>\r\n\t </div>\r\n \t<div class="col-auto">\r\n \t\t\t\t<button type="button" class="btn btn-primary" onclick="clearForm(); return false;">Clear</button>\r\n\t </div>\r\n </div>\r\n\t\t\t \t </div>\r\n <script>\r\n\t\tdocument.getElementById("filter-search").addEventListener("keyup", function(event) { event.preventDefault(); if (event.keyCode === 13) { document.getElementById("searchbtn").click(); } });\r\n </script>\r\n\t\t\t\t\r\n\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t</div>\r\n\t\t\r\n\t\t\t\t\t<div class="latestnews-items">\r\n\t\t\t\t <div class="latestnews-item id-368 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/368-pierre-bellec" class="hasTooltip" title="Pierre Bellec" aria-label="Read more about Pierre Bellec"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_368.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Pierre Bellec" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/368-pierre-bellec" class="hasTooltip" title="Pierre Bellec" aria-label="Read more about Pierre Bellec"> <span>Pierre Bellec</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:pcb71@stat.rutgers.edu">pcb71@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-715 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/715-matteo-bonvini" class="hasTooltip" title="Matteo Bonvini" aria-label="Read more about Matteo Bonvini"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_715.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Matteo Bonvini" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/715-matteo-bonvini" class="hasTooltip" title="Matteo Bonvini" aria-label="Read more about Matteo Bonvini"> <span>Matteo Bonvini</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mb1662@stat.rutgers.edu">mb1662@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-369 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/369-steven-buyske" class="hasTooltip" title="Steve Buyske" aria-label="Read more about Steve Buyske"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_369.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Steve Buyske" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/369-steven-buyske" class="hasTooltip" title="Steve Buyske" aria-label="Read more about Steve Buyske"> <span>Steve Buyske</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor; Co-Director of Undergraduate Statistics Program; Co-Director of Undergraduate Data Science Program</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:buyske@stat.rutgers.edu">buyske@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-370 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/370-javier-cabrera" class="hasTooltip" title="Javier Cabrera" aria-label="Read more about Javier Cabrera"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_370.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Javier Cabrera" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/370-javier-cabrera" class="hasTooltip" title="Javier Cabrera" aria-label="Read more about Javier Cabrera"> <span>Javier Cabrera</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:cabrera@stat.rutgers.edu">cabrera@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-371 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/371-rong-chen" class="hasTooltip" title="Rong Chen" aria-label="Read more about Rong Chen"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_371.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Rong Chen" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/371-rong-chen" class="hasTooltip" title="Rong Chen" aria-label="Read more about Rong Chen"> <span>Rong Chen</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Chair</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:rongchen@stat.rutgers.edu">rongchen@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-650 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/650-yaqing-chen" class="hasTooltip" title="Yaqing Chen" aria-label="Read more about Yaqing Chen"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_650.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Yaqing Chen" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/650-yaqing-chen" class="hasTooltip" title="Yaqing Chen" aria-label="Read more about Yaqing Chen"> <span>Yaqing Chen</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:yqchen@stat.rutgers.edu">yqchen@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-373 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/373-harry-crane" class="hasTooltip" title="Harry Crane" aria-label="Read more about Harry Crane"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_373.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Harry Crane" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/373-harry-crane" class="hasTooltip" title="Harry Crane" aria-label="Read more about Harry Crane"> <span>Harry Crane</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:hcrane@stat.rutgers.edu">hcrane@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-374 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/374-tirthankar-dasgupta" class="hasTooltip" title="Tirthankar DasGupta" aria-label="Read more about Tirthankar DasGupta"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_374.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Tirthankar DasGupta" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/374-tirthankar-dasgupta" class="hasTooltip" title="Tirthankar DasGupta" aria-label="Read more about Tirthankar DasGupta"> <span>Tirthankar DasGupta</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Graduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:tirthankar.dasgupta@rutgers.edu">tirthankar.dasgupta@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-403 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/403-ruobin-gong" class="hasTooltip" title="Ruobin Gong" aria-label="Read more about Ruobin Gong"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_403.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Ruobin Gong" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/403-ruobin-gong" class="hasTooltip" title="Ruobin Gong" aria-label="Read more about Ruobin Gong"> <span>Ruobin Gong</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ruobin.gong@rutgers.edu">ruobin.gong@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-400 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/400-zijian-guo" class="hasTooltip" title="Zijian Guo" aria-label="Read more about Zijian Guo"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_400.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Zijian Guo" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/400-zijian-guo" class="hasTooltip" title="Zijian Guo" aria-label="Read more about Zijian Guo"> <span>Zijian Guo</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:zijguo@stat.rutgers.edu">zijguo@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-399 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/399-qiyang-han" class="hasTooltip" title="Qiyang Han" aria-label="Read more about Qiyang Han"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_399.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Qiyang Han" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/399-qiyang-han" class="hasTooltip" title="Qiyang Han" aria-label="Read more about Qiyang Han"> <span>Qiyang Han</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:qh85@stat.rutgers.edu">qh85@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-398 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/398-donald-r-hoover" class="hasTooltip" title="Donald R. Hoover" aria-label="Read more about Donald R. Hoover"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_398.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Donald R. Hoover" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/398-donald-r-hoover" class="hasTooltip" title="Donald R. Hoover" aria-label="Read more about Donald R. Hoover"> <span>Donald R. Hoover</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:drhoover@stat.rutgers.edu">drhoover@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-397 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/397-ying-hung" class="hasTooltip" title="Ying Hung" aria-label="Read more about Ying Hung"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_397.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Ying Hung" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/397-ying-hung" class="hasTooltip" title="Ying Hung" aria-label="Read more about Ying Hung"> <span>Ying Hung</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:yhung@stat.rutgers.edu">yhung@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-651 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/651-koulik-khamaru" class="hasTooltip" title="Koulik Khamaru" aria-label="Read more about Koulik Khamaru"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_651.jpeg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Koulik Khamaru" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/651-koulik-khamaru" class="hasTooltip" title="Koulik Khamaru" aria-label="Read more about Koulik Khamaru"> <span>Koulik Khamaru</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:kk1241@stat.rutgers.edu">kk1241@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-395 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/395-john-kolassa" class="hasTooltip" title="John Kolassa" aria-label="Read more about John Kolassa"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_395.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="John Kolassa" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/395-john-kolassa" class="hasTooltip" title="John Kolassa" aria-label="Read more about John Kolassa"> <span>John Kolassa</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:kolassa@stat.rutgers.edu">kolassa@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-391 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/391-regina-y-liu" class="hasTooltip" title="Regina Y. Liu" aria-label="Read more about Regina Y. Liu"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_391.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Regina Y. Liu" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/391-regina-y-liu" class="hasTooltip" title="Regina Y. Liu" aria-label="Read more about Regina Y. Liu"> <span>Regina Y. Liu</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:rliu@stat.rutgers.edu">rliu@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-716 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/716-gemma-moran" class="hasTooltip" title="Gemma Moran" aria-label="Read more about Gemma Moran"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_716.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Gemma Moran" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/716-gemma-moran" class="hasTooltip" title="Gemma Moran" aria-label="Read more about Gemma Moran"> <span>Gemma Moran</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:gm845@stat.rutgers.edu">gm845@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-573 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/573-nicole-pashley" class="hasTooltip" title="Nicole Pashley" aria-label="Read more about Nicole Pashley"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_573.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Nicole Pashley" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/573-nicole-pashley" class="hasTooltip" title="Nicole Pashley" aria-label="Read more about Nicole Pashley"> <span>Nicole Pashley</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:np755@stat.rutgers.edu">np755@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-387 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/387-harold-b-sackrowitz" class="hasTooltip" title="Harold B. Sackrowitz" aria-label="Read more about Harold B. Sackrowitz"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_387.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Harold B. Sackrowitz" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/387-harold-b-sackrowitz" class="hasTooltip" title="Harold B. Sackrowitz" aria-label="Read more about Harold B. Sackrowitz"> <span>Harold B. Sackrowitz</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Undergraduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:sackrowi@stat.rutgers.edu">sackrowi@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-542 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/542-michael-l-stein" class="hasTooltip" title="Michael L. Stein" aria-label="Read more about Michael L. Stein"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_542.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Michael L. Stein" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/542-michael-l-stein" class="hasTooltip" title="Michael L. Stein" aria-label="Read more about Michael L. Stein"> <span>Michael L. Stein</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ms2870@stat.rutgers.edu">ms2870@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-383 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/383-zhiqiang-tan" class="hasTooltip" title="Zhiqiang Tan" aria-label="Read more about Zhiqiang Tan"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_383.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Zhiqiang Tan" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/383-zhiqiang-tan" class="hasTooltip" title="Zhiqiang Tan" aria-label="Read more about Zhiqiang Tan"> <span>Zhiqiang Tan</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor </span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:ztan@stat.rutgers.edu">ztan@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-382 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/382-david-e-tyler" class="hasTooltip" title="David E. Tyler" aria-label="Read more about David E. Tyler"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_382.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="David E. Tyler" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/382-david-e-tyler" class="hasTooltip" title="David E. Tyler" aria-label="Read more about David E. Tyler"> <span>David E. Tyler</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:dtyler@stat.rutgers.edu">dtyler@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-572 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/572-guanyang-wang" class="hasTooltip" title="Guanyang Wang" aria-label="Read more about Guanyang Wang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_572.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Guanyang Wang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/572-guanyang-wang" class="hasTooltip" title="Guanyang Wang" aria-label="Read more about Guanyang Wang"> <span>Guanyang Wang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:guanyang.wang@rutgers.edu">guanyang.wang@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-381 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/381-sijan-wang" class="hasTooltip" title="Sijian Wang" aria-label="Read more about Sijian Wang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_381.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Sijian Wang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/381-sijan-wang" class="hasTooltip" title="Sijian Wang" aria-label="Read more about Sijian Wang"> <span>Sijian Wang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Director of FSRM and MSDS programs</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:sijian.wang@stat.rutgers.edu">sijian.wang@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-380 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/380-han-xiao" class="hasTooltip" title="Han Xiao" aria-label="Read more about Han Xiao"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_380.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Han Xiao" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/380-han-xiao" class="hasTooltip" title="Han Xiao" aria-label="Read more about Han Xiao"> <span>Han Xiao</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Professor and Co-Graduate Director</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:hxiao@stat.rutgers.edu">hxiao@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-379 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/379-minge-xie" class="hasTooltip" title="Minge Xie" aria-label="Read more about Minge Xie"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_379.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Minge Xie" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/379-minge-xie" class="hasTooltip" title="Minge Xie" aria-label="Read more about Minge Xie"> <span>Minge Xie</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Director, Office of Statistical Consulting</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mxie@stat.rutgers.edu">mxie@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-378 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/378-min-xu" class="hasTooltip" title="Min Xu" aria-label="Read more about Min Xu"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_378.jpg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Min Xu" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/378-min-xu" class="hasTooltip" title="Min Xu" aria-label="Read more about Min Xu"> <span>Min Xu</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Assistant Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:mx76@stat.rutgers.edu">mx76@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-376 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/376-cun-hui-zhang" class="hasTooltip" title="Cun-Hui Zhang" aria-label="Read more about Cun-Hui Zhang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_376.png?3088073d1e73eeccbd4c2a2efb7f218f" alt="Cun-Hui Zhang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/376-cun-hui-zhang" class="hasTooltip" title="Cun-Hui Zhang" aria-label="Read more about Cun-Hui Zhang"> <span>Cun-Hui Zhang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Distinguished Professor and Co-Director of FSRM and MSDS programs</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:czhang@stat.rutgers.edu">czhang@stat.rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> <div class="latestnews-item id-546 catid-130 text_bottom"> <div class="news"> <div class="innernews"> <div class="newshead picturetype"> <div class="picture"> <div class="innerpicture"> <a href="/people-pages/faculty/people/546-linjun-zhang" class="hasTooltip" title="Linjun Zhang" aria-label="Read more about Linjun Zhang"> <img src="/media/cache/com_latestnewsenhancedpro/thumb_articles_blog_100570_546.jpeg?3088073d1e73eeccbd4c2a2efb7f218f" alt="Linjun Zhang" width="300" height="300" loading="eager"> </a> </div> </div> </div> <div class="newsinfo"> <h2 class="newstitle "> <a href="/people-pages/faculty/people/546-linjun-zhang" class="hasTooltip" title="Linjun Zhang" aria-label="Read more about Linjun Zhang"> <span>Linjun Zhang</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5 "><span class="detail_data"><a href="mailto:linjun.zhang@rutgers.edu">linjun.zhang@rutgers.edu</a></span></span></dd></dl> </div> </div> </div> </div> \t\t\t</div>\r\n\t\t\r\n\t\t<div class="pagination_wrapper bottom">\r\n\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t<div class="counterpagination">\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t</div>\r\n\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t\t</div>\r\n\t</form>\r\n\r\n\t</div>\r\n\t<div id="lnepmodal" data-backdrop="false" data-keyboard="true" data-remote="true" class="modal fade" tabindex="-1" role="dialog" aria-labelledby="lnepmodalLabel" aria-hidden="true">\r\n \t<div class="modal-dialog" role="document">\r\n \t\t<div class="modal-content">\r\n \t<div class="modal-header">\r\n \t\t \t\t \t\t \t\t\t<h5 id="lnepmodalLabel" class="modal-title">Article</h5>\r\n \t\t\t<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>\r\n \t\t \t</div>\r\n \t<div class="modal-body">\r\n \t\t<iframe class="iframe" height="500" style="display: block; width: 100%; border: 0; max-height: none; overflow: auto"></iframe>\r\n \t</div>\r\n \t<div class="modal-footer">\r\n \t\t<button class="btn btn-secondary" data-bs-dismiss="modal" aria-hidden="true">Close</button>\r\n \t</div>\r\n \t\t</div>\r\n \t</div>\r\n </div>\n\t\t\t</main>\n \t\t \t\t \t\t\t\t</div>\n\n\t\t\n\t\t\n\t\t \n \n \t\t \n \t\t\t</div>\n\n\t \n \n\t\n\t \n \n \t\t\t<sas-footer-identity class=" grid-child container-sas-footer-identity full-width-v2">\n\t\t\t<div class=" sas-footer-identity">\n\t\t\t\t<div class="sas-footer-identity no-card sas-footer-logo-left sas-footer-logo-left sas-branding">\n \n<div id="mod-custom261" class="mod-custom custom">\n <p><img src="/media/templates/site/cassiopeia_sas/images/RNBSAS_H_WHITE.svg" alt="White RU Logo" style="min-width: 300px; max-width: 400px; margin-top: 10px; margin-left: 15px; margin-bottom: 10px;" loading="lazy" /></p></div>\n</div>\n<div class="sas-footer-identity no-card ">\n <ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list mod-menu mod-list nav navbar-sas-ru sas-footer-menu sas-footer-menu-right">\n<li class="metismenu-item item-101205 level-1"><a href="https://sas.rutgers.edu/about/events/upcoming-events" target="_blank" rel="noopener noreferrer">SAS Events</a></li><li class="metismenu-item item-101206 level-1"><a href="https://sas.rutgers.edu/about/news" target="_blank" rel="noopener noreferrer">SAS News</a></li><li class="metismenu-item item-100002 level-1"><a href="https://www.rutgers.edu" target="_blank" rel="noopener noreferrer">rutgers.edu</a></li><li class="metismenu-item item-100118 level-1"><a href="https://sas.rutgers.edu" target="_blank" rel="noopener noreferrer">SAS</a></li><li class="metismenu-item item-100009 level-1"><a href="https://search.rutgers.edu/people" target="_blank" rel="noopener noreferrer">Search People</a></li><li class="metismenu-item item-100010 level-1"><a href="/search-website" ><img src="/media/templates/site/cassiopeia_sas/images/search-magnifying-glass.PNG" alt="" width="25" height="24" loading="lazy"><span class="image-title visually-hidden">Search Website</span></a></li></ul>\n</div>\n\n\t\t\t</div>\n\t\t</sas-footer-identity>\n\t\n<footer class="container-footer footer full-width">\n \n \n <!-- Display any module in the footer position from the sites --> \n<div class="grid-child">\n<!--2025-01 LG: Display Rutgers Menu from rutgers file --> \n<div><h3 class="title">Connect with Rutgers</h3>\n<ul class="list-unstyled">\n<li><a href="https://newbrunswick.rutgers.edu/" target="_blank" >Rutgers New Brunswick</a>\n<li><a href="https://www.rutgers.edu/news" target="blank">Rutgers Today</a>\n<li><a href="https://my.rutgers.edu/uxp/login" target="_blank">myRutgers</a>\n<li><a href="https://scheduling.rutgers.edu/scheduling/academic-calendar" target="_blank">Academic Calendar</a>\n<li><a href="https://classes.rutgers.edu//soc/#home" target="_blank">Rutgers Schedule of Classes</a>\n<li><a href="https://emnb.rutgers.edu/one-stop-overview/" target="_blank">One Stop Student Service Center</a>\n<li><a href="https://rutgers.campuslabs.com/engage/events/" target="_blank">getINVOLVED</a>\n<li><a href="https://admissions.rutgers.edu/visit-rutgers" target="_blank">Plan a Visit</a>\n</ul>\n</div> \n <!--2025-01 LG: Display SAS Menu from SAS file --> \n <div>\n <h3 class="title">Explore SAS</h3>\n<ul class="list-unstyled">\n<li><a href="https://sas.rutgers.edu/academics/majors-minors" target="blank">Majors and Minors</a>\n<li><a href="https://sas.rutgers.edu/academics/areas-of-study" target="_blank" >Departments and Programs</a>\n<li><a href="https://sas.rutgers.edu/academics/centers-institutes" target="_blank">Research Centers and Institutes</a>\n<li><a href="https://sas.rutgers.edu/about/sas-offices" target="_blank">SAS Offices</a> \n<li><a href="https://sas.rutgers.edu/giving" target="_blank">Support SAS</a>\n</ul>\n</div> \n \n<!--2025-01 LG: Display NOTICES Menu from NOTICES file --> \n<div>\n<h3 class="title">Notices</h3>\n<ul class="list-unstyled">\n<li><a href="https://www.rutgers.edu/status" target="_blank" >University Operating Status</a>\n</ul>\n<hr>\n<ul class="list-unstyled">\n<li><a href="https://www.rutgers.edu/privacy-statement" target="blank">Privacy</a>\n</ul>\n</div> \n <div class="moduletable ">\n <h3 class="title">Contact Us</h3> \n<div id="mod-custom96" class="mod-custom custom">\n <p><img src="/images/stories/hill2_70x70.jpg" alt="hill2_70x70" width="90" height="90" style="margin-right: 10px; margin-bottom: 5px; margin-top: 10px; float: left;" />501 Hill Center<br />110 Frelinghuysen Road<br />Piscataway, NJ 08854<br /><strong>_____________________________</strong><br />Phone: 848-445-2690</p>\r\n<p>General Inquiries: <joomla-hidden-mail is-link="1" is-email="1" first="YWRtaW4=" last="c3RhdC5ydXRnZXJzLmVkdQ==" text="YWRtaW5Ac3RhdC5ydXRnZXJzLmVkdQ==" base="" >This email address is being protected from spambots. You need JavaScript enabled to view it.</joomla-hidden-mail><br />Special Permission: <a href="https://secure.sas.rutgers.edu/apps/special_permission/" target="_blank" rel="noopener">https://secure.sas.rutgers.edu/apps/special_permission/</a><br /><joomla-hidden-mail is-link="1" is-email="1" first="ZXNoYXJrZXk=" last="c3RhdC5ydXRnZXJzLmVkdQ==" text="ZXNoYXJrZXlAc3RhdC5ydXRnZXJzLmVkdQ==" base="" >This email address is being protected from spambots. You need JavaScript enabled to view it.</joomla-hidden-mail></p></div>\n</div>\n\n\t</div>\n</footer>\n\n<!-- Display Social Media Module --> \n\t \n\n<!--Display Footer Menu --> \n \n\t\t\t<sas-footer-menu class="container-sas-footer-menu full-width">\n\t\t\t<div class="sas-footer-menu">\n\t\t\t\t<ul class="mod-menu mod-menu_dropdown-metismenu metismenu mod-list navbar navbar-nav dropdown sas-footer-menu">\n<li class="metismenu-item item-100003 level-1"><a href="/" >Home</a></li><li class="metismenu-item item-100052 level-1"><a href="https://ithelp.sas.rutgers.edu/" target="_blank" rel="noopener noreferrer">IT Help</a></li><li class="metismenu-item item-100119 level-1"><a href="/contact-us" >Contact Us</a></li><li class="metismenu-item item-100639 level-1"><a href="/site-map?view=html&id=2" >Site Map</a></li><li class="metismenu-item item-100827 level-1"><a href="/search-in-footer" >Search</a></li><li class="metismenu-item item-100772 level-1"><a href="https://statistics.rutgers.edu/?morequest=sso&idp=urn:mace:incommon:rutgers.edu" >Login</a></li></ul>\n\n\t\t\t</div>\n\t\t</sas-footer-menu>\n\t\n\n<!-- Display Copyright -->\n\t\t<copyright class="container-sas-copyright full-width">\n\t\t\t<div class="sas-copyright">\n\n<!--2025-01 LG: Display Copyright Text from copyright text file --> \n<p>\n <!-- paragraph 1 of copy right - information -->\n<p style="text-align: center;">Rutgers is an equal access/equal opportunity institution. Individuals with disabilities are encouraged to direct suggestions, comments, or complaints concerning any<br />\naccessibility issues with Rutgers websites to <a href=\'mailto:accessibility@rutgers.edu\'>accessibility@rutgers.edu</a> or complete the <a href=\'https://it.rutgers.edu/it-accessibility-initiative/barrierform/\' rel=\'nofollow\' target=\'_blank\'>Report Accessibility Barrier / Provide Feedback</a> form.</p>\n <!-- paragraph 2 of copy right - information -->\n<p style="text-align: center;"><a href=\'https://www.rutgers.edu/copyright-information\' rel=\'nofollow\' target=\'_blank\'>Copyright ©<script>document.write(new Date().getFullYear())</script></a>, <a href=\'https://www.rutgers.edu/\' rel=\'nofollow\' target=\'_blank\'>Rutgers, The State University of New Jersey</a>. All rights reserved. <a href=\'https://ithelp.sas.rutgers.edu/\' rel=\'nofollow\' target=\'_blank\'>Contact webmaster</a></p>\n<p> </p>\n\n</p>\n\n<!-- Display back to top link --> \n\t\t\t<a href="#top" id="back-top" class="back-to-top-link" aria-label="Back to Top">\n\t\t\t<span class="icon-arrow-up icon-fw" aria-hidden="true"></span>\n\t\t</a>\n\n\t\n\n<noscript class="4SEO_cron">\n <img aria-hidden="true" alt="" style="position:absolute;bottom:0;left:0;z-index:-99999;" src="https://statistics.rutgers.edu/index.php/_wblapi?nolangfilter=1&_wblapi=/forseo/v1/cron/image/" data-pagespeed-no-transform data-speed-no-transform />\n</noscript>\n<script class="4SEO_cron" data-speed-no-transform >setTimeout(function () {\n var e = document.createElement(\'img\');\n e.setAttribute(\'style\', \'position:absolute;bottom:0;right:0;z-index:-99999\');\n e.setAttribute(\'aria-hidden\', \'true\');\n e.setAttribute(\'src\', \'https://statistics.rutgers.edu/index.php/_wblapi?nolangfilter=1&_wblapi=/forseo/v1/cron/image/\' + Math.random().toString().substring(2) + Math.random().toString().substring(2) + \'.svg\');\n document.body.appendChild(e);\n setTimeout(function () {\n document.body.removeChild(e)\n }, 3000)\n }, 3000);\n</script>\n</body>\n</html>\n'
Websites store and transfer text using character encodings, which determine how characters are represented as bytes. Websites generally specify their encoding in the HTML Meta tags, or in HTTP headers.
html
<meta charset="UTF-8">
Unicode defines which characters exist and assigns them numbers. UTF-8 is one of several encoding schemes (including UTF-16 and UTF-32) that determine how those numbers are stored in a computer.
We use Beautiful Soup
to represent the HTML as a nested data structure:
= bs4.BeautifulSoup(response.text, "html.parser")
soup print(soup.prettify()[:500])
<!DOCTYPE html>
<html dir="ltr" lang="en-gb">
<head>
<meta charset="utf-8"/>
<meta content="width=device-width, initial-scale=1" name="viewport"/>
<meta content="The School of Arts and Sciences, Rutgers, The State University of New Jersey" name="description"/>
<meta content="Joomla! - Open Source Content Management" name="generator"/>
<title>
Faculty
</title>
<link href="/media/templates/site/cassiopeia_sas/images/favicon.ico" rel="alternate icon" type="image/vnd.microsoft.icon
soup.title
<title>Faculty</title>
soup.title.name
'title'
soup.title.string
'Faculty'
By inspecting the webpage with devtools in a browser, we see that the information is in nodes with CSS class newsinfo
. We can access it using Beautiful Soup’s find_all
method (more details on find_all
here).
= soup.find_all(class_="newsinfo")
faculty_items 0] faculty_items[
<div class="newsinfo"> <h2 class="newstitle"> <a aria-label="Read more about Pierre Bellec" class="hasTooltip" href="/people-pages/faculty/people/368-pierre-bellec" title="Pierre Bellec"> <span>Pierre Bellec</span> </a> </h2> <dl class="item_details before_text"><dt>Information</dt><dd class="newsextra"><span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm"><span class="detail_data">Associate Professor</span></span></dd><dd class="newsextra"><span class="detail detail_jfield_url detail_jfield_5"><span class="detail_data"><a href="mailto:pcb71@stat.rutgers.edu">pcb71@stat.rutgers.edu</a></span></span></dd></dl> </div>
print(faculty_items[0].prettify())
<div class="newsinfo">
<h2 class="newstitle">
<a aria-label="Read more about Pierre Bellec" class="hasTooltip" href="/people-pages/faculty/people/368-pierre-bellec" title="Pierre Bellec">
<span>
Pierre Bellec
</span>
</a>
</h2>
<dl class="item_details before_text">
<dt>
Information
</dt>
<dd class="newsextra">
<span class="detail detail_jfield_text detail_jfield_2 caps-gray-sm">
<span class="detail_data">
Associate Professor
</span>
</span>
</dd>
<dd class="newsextra">
<span class="detail detail_jfield_url detail_jfield_5">
<span class="detail_data">
<a href="mailto:pcb71@stat.rutgers.edu">
pcb71@stat.rutgers.edu
</a>
</span>
</span>
</dd>
</dl>
</div>
We can see that the faculty name is in the first span. Note: .find
gets only the first instance (unlike .find_all
which gets all instances).
0].find("span") faculty_items[
<span>Pierre Bellec</span>
or to get the text of the node:
0].find("span").text faculty_items[
'Pierre Bellec'
The title is in a span tag with class detail_jfield_2
:
0].find(class_="detail_jfield_2").text faculty_items[
'Associate Professor'
Finally, the email is given in a span tag with class detail_jfield_5
:
0].find("span", class_="detail_jfield_5").text faculty_items[
'pcb71@stat.rutgers.edu'
Let’s turn this into a pandas DataFrame:
# create dictionary of data
= []
data
for item in faculty_items:
'name': item.find("span").text,
data.append({'title': item.find("span", class_='detail_jfield_2').text,
'email': item.find("span", class_='detail_jfield_5').text})
# turn into pandas DataFrame
pd.DataFrame(data)
name | title | ||
---|---|---|---|
0 | Pierre Bellec | Associate Professor | pcb71@stat.rutgers.edu |
1 | Matteo Bonvini | Assistant Professor | mb1662@stat.rutgers.edu |
2 | Steve Buyske | Associate Professor; Co-Director of Undergradu... | buyske@stat.rutgers.edu |
3 | Javier Cabrera | Professor | cabrera@stat.rutgers.edu |
4 | Rong Chen | Distinguished Professor and Chair | rongchen@stat.rutgers.edu |
5 | Yaqing Chen | Assistant Professor | yqchen@stat.rutgers.edu |
6 | Harry Crane | Professor | hcrane@stat.rutgers.edu |
7 | Tirthankar DasGupta | Professor and Co-Graduate Director | tirthankar.dasgupta@rutgers.edu |
8 | Ruobin Gong | Associate Professor | ruobin.gong@rutgers.edu |
9 | Zijian Guo | Associate Professor | zijguo@stat.rutgers.edu |
10 | Qiyang Han | Associate Professor | qh85@stat.rutgers.edu |
11 | Donald R. Hoover | Professor | drhoover@stat.rutgers.edu |
12 | Ying Hung | Professor | yhung@stat.rutgers.edu |
13 | Koulik Khamaru | Assistant Professor | kk1241@stat.rutgers.edu |
14 | John Kolassa | Distinguished Professor | kolassa@stat.rutgers.edu |
15 | Regina Y. Liu | Distinguished Professor | rliu@stat.rutgers.edu |
16 | Gemma Moran | Assistant Professor | gm845@stat.rutgers.edu |
17 | Nicole Pashley | Assistant Professor | np755@stat.rutgers.edu |
18 | Harold B. Sackrowitz | Distinguished Professor and Undergraduate Dire... | sackrowi@stat.rutgers.edu |
19 | Michael L. Stein | Distinguished Professor | ms2870@stat.rutgers.edu |
20 | Zhiqiang Tan | Distinguished Professor | ztan@stat.rutgers.edu |
21 | David E. Tyler | Distinguished Professor | dtyler@stat.rutgers.edu |
22 | Guanyang Wang | Assistant Professor | guanyang.wang@rutgers.edu |
23 | Sijian Wang | Professor and Co-Director of FSRM and MSDS pro... | sijian.wang@stat.rutgers.edu |
24 | Han Xiao | Professor and Co-Graduate Director | hxiao@stat.rutgers.edu |
25 | Minge Xie | Distinguished Professor and Director, Office o... | mxie@stat.rutgers.edu |
26 | Min Xu | Assistant Professor | mx76@stat.rutgers.edu |
27 | Cun-Hui Zhang | Distinguished Professor and Co-Director of FSR... | czhang@stat.rutgers.edu |
28 | Linjun Zhang | Associate Professor | linjun.zhang@rutgers.edu |
NOTE: In Lecture 4, we used pd.read_html
to get tables from HTML. Often the data we want is not in a table, however, like the Rutgers website.
Selector Gadget
In the previous example, we went through the HTML to see what class we needed to use.
Fortunately, there are tools available to make this process easier.
SelectorGadget is an open-source tool that simplifies identifying the generating and finding of CSS selectors.
Installation
- To install it, open this page in your browser, and then drag the link to your bookmark bar
Usage
- Open the page you want to scrape
- Click SelectorGadget in your bookmark bar
- Click on the element you want to select
- Unclick elements you don’t want selected
- Copy CSS selectors
CSS Selectors
The CSS selectors that Selector Gadget gives you follow certain rules. Here is a short summary of some CSS selector rules (source).
Selector passed to the select() method | Will match . . . |
---|---|
soup.select('div') |
All elements named <div> |
soup.select('#author') |
The element with an id attribute of author |
soup.select('.notice') |
All elements that use a CSS class attribute named notice |
soup.select('div span') |
All elements named <span> that are within an element named <div> |
soup.select('div > span') |
All elements named <span> that are directly within an element named <div> , with no other element in between |
soup.select('input[name]') |
All elements named <input> that have a name attribute with any value |
soup.select('input[type="button"]') |
All elements named <input> that have an attribute named type with value button |
Here is a fun tutorial to practice CSS selector rules: CSS dinner.
Example: Rutgers MSDS Courses
For example, suppose we want to scrape the MSDS course website. We can use Selector Gadget to click on the items we are interested in. In the bottom right corner, Selector Gadget will display the HTML element or class we need.
In this case, the titles are the h2
element.
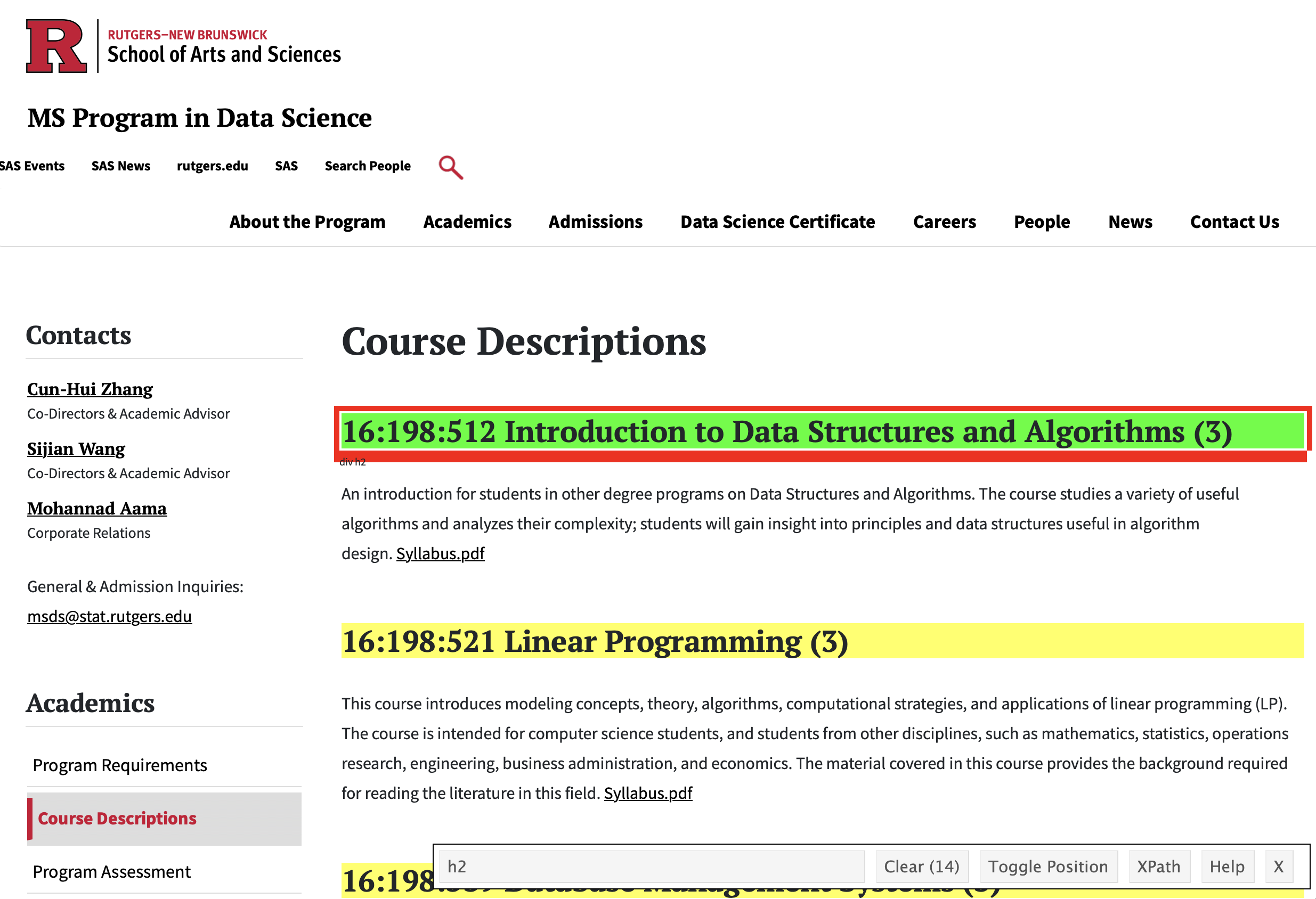
= "https://msds-stat.rutgers.edu/msds-academics/msds-coursedesc"
url = requests.get(url)
response response
<Response [200]>
= bs4.BeautifulSoup(response.text, "html.parser") soup
The function soup.select
runs a CSS selector against a parsed document and returns all the matching elements. We use soup.select()
to extract the CSS selector h2
.
= soup.select("h2") elements
0] elements[
<h2>
16:198:512 Introduction to Data Structures and Algorithms (3) </h2>
To get rid of the extra space, we can use the .strip()
string method (documentation here).
= elements[0].text.strip()
course course
'16:198:512 Introduction to Data Structures and Algorithms (3)'
Using string methods such as .split
and .join
, we can extract:
- the course code
- the number of credits
' ') course.split(
['16:198:512',
'Introduction',
'to',
'Data',
'Structures',
'and',
'Algorithms',
'(3)']
= course.split(' ')[0]
code code
'16:198:512'
= course[-2:-1]
credit credit
'3'
= course.split(' ')[1:-1]
name = (' ').join(name)
name name
'Introduction to Data Structures and Algorithms'
Going back to Selector Gadget, we can also see that the course descriptions are .item-content p
.
= soup.select('.item-content p') descriptions
0] descriptions[
<p>An introduction for students in other degree programs on Data Structures and Algorithms. The course studies a variety of useful algorithms and analyzes their complexity; students will gain insight into principles and data structures useful in algorithm design. <a class="wf_file" href="/images/Home/syllabus/198_512.pdf">Syllabus.pdf</a></p>
0].text descriptions[
'An introduction for students in other degree programs on Data Structures and Algorithms. The course studies a variety of useful algorithms and analyzes their complexity; students will gain insight into principles and data structures useful in algorithm design.\xa0Syllabus.pdf'
We can see everything in the <a>
tag has turned into the Unicode symbol \xa0
followed by the text.
0].text.split('\xa0')[0] descriptions[
'An introduction for students in other degree programs on Data Structures and Algorithms. The course studies a variety of useful algorithms and analyzes their complexity; students will gain insight into principles and data structures useful in algorithm design.'
= [i.text.split('\xa0')[0] for i in descriptions] descriptions
= [
courses 'title': ' '.join(element.text.strip().split(' ')[1:-1]),
{'course_number': element.text.strip().split(' ')[0],
'credits': element.text.strip()[-2:-1],
}for element in elements
]
= pd.DataFrame(courses)
courses_df 'description'] = descriptions
courses_df[ courses_df
title | course_number | credits | description | |
---|---|---|---|---|
0 | Introduction to Data Structures and Algorithms | 16:198:512 | 3 | An introduction for students in other degree p... |
1 | Linear Programming | 16:198:521 | 3 | This course introduces modeling concepts, theo... |
2 | Database Management Systems | 16:198:539 | 3 | Implementing components of relational database... |
3 | Advanced Database Management | 16:198:541 | 3 | This course focuses on advanced topics in Data... |
4 | Convex Optimization for Engineering Applications | 16:332:509 | 3 | The course develops the necessary theory, algo... |
5 | Statistical Learning for Data Science | 16:954:534 | 3 | Advanced statistical learning methods are esse... |
6 | Statistical Models and Computing | 16:954:567 | 3 | This course is about advanced statistical mode... |
7 | Advanced Analytics using Statistical Software | 16:954:577 | 3 | Modeling and analysis of data, usually very la... |
8 | Probability and Statistical Inference for Data... | 16:954:581 | 3 | The study of probabilistic and inferential too... |
9 | Regression and Time Series Analysis for Data S... | 16:954:596 | 3 | This course introduces regression methods, sta... |
10 | Data Wrangling and Husbandry | 16:954:597 | 3 | This course provides an introduction to the pr... |
11 | Advanced Simulation Methods | 16:958:587 | 3 | The emphasis of this course will be on Modern ... |
12 | Financial Data Mining | 16:958:588 | 3 | Databases and data warehousing, exploratory da... |
13 | Advanced Programming for Financial Statistics ... | 16:958:589 | 3 | This course covers the basic concepts of objec... |
APIs
Many website have an application programming interface (API).
An API consists of programmatic instructions for how to interact with a piece of software.
APIs allow for website owners to control who has access to data, what data they can access, and how much of the data.
Aspect | Web scraping | APIs |
---|---|---|
Availability | Can extract data from any public website | Only websites with APIs |
Access | Can be blocked by anti-bot systems | Restrictions based on usage or paid plan limitations |
Data Format | Raw HTML | Often JSON/XML data |
Speed | Slower for large-scale scraping | Faster as data directly available |
Stability | Depends on website structure changes | Generally more stable |
Technical setup | Custom per website | Usually straightforward |
Legality | Need careful consideration | Clear terms and conditions |
Table adapted from here.
Example: NASA API
This example is adapted from Data Science: A First Introduction with Python.
We will access NASA’s “Astronomy Picture of the Day”.
- Go to NASA’s API page and generate an API key. NOTE: we are limited to 1000 requests per hour.
- Save your API key in
nasa_api.txt
. - The NASA API is what is known as an HTTP API. This is a common kind of API where you can access data using a particular URL.
HTTP API
HTTP APIs consist of:
- URL endpoint e.g.
https://api.nasa.gov/planetary/apod
?
which denotes that query parameters will follow- parameters of the form
parameter=value
, separated by&
- Let’s get the data!
with open('nasa_api.txt', 'r') as file:
= file.read().strip() API_KEY
='2025-03-26' date
= 'https://api.nasa.gov/planetary/apod' +\
nasa_url '?' +\
'api_key=' +\
+\
API_KEY '&' +\
'date=' +\
date
= requests.get(nasa_url) nasa_data_single
nasa_data_single.json()
{'copyright': '\nJuan Montilla \n(AAE)\n',
'date': '2025-03-26',
'explanation': "You'd think the Pacman Nebula would be eating stars, but actually it is forming them. Within the nebula, a cluster's young, massive stars are powering the pervasive nebular glow. The eye-catching shapes looming in the featured portrait of NGC 281 are sculpted dusty columns and dense Bok globules seen in silhouette, eroded by intense, energetic winds and radiation from the hot cluster stars. If they survive long enough, the dusty structures could also be sites of future star formation. Playfully called the Pacman Nebula because of its overall shape, NGC 281 is about 10,000 light-years away in the constellation Cassiopeia. This sharp composite image was made through narrow-band filters in Spain in mid 2024. It combines emissions from the nebula's hydrogen and oxygen atoms to synthesize red, green, and blue colors. The scene spans well over 80 light-years at the estimated distance of NGC 281.",
'hdurl': 'https://apod.nasa.gov/apod/image/2503/Pacman_Montilla_1500.jpg',
'media_type': 'image',
'service_version': 'v1',
'title': 'Star Formation in the Pacman Nebula',
'url': 'https://apod.nasa.gov/apod/image/2503/Pacman_Montilla_1080.jpg'}
from IPython.display import Image, display
= nasa_data_single.json()['url']
image_url =image_url, width=600)) display(Image(url
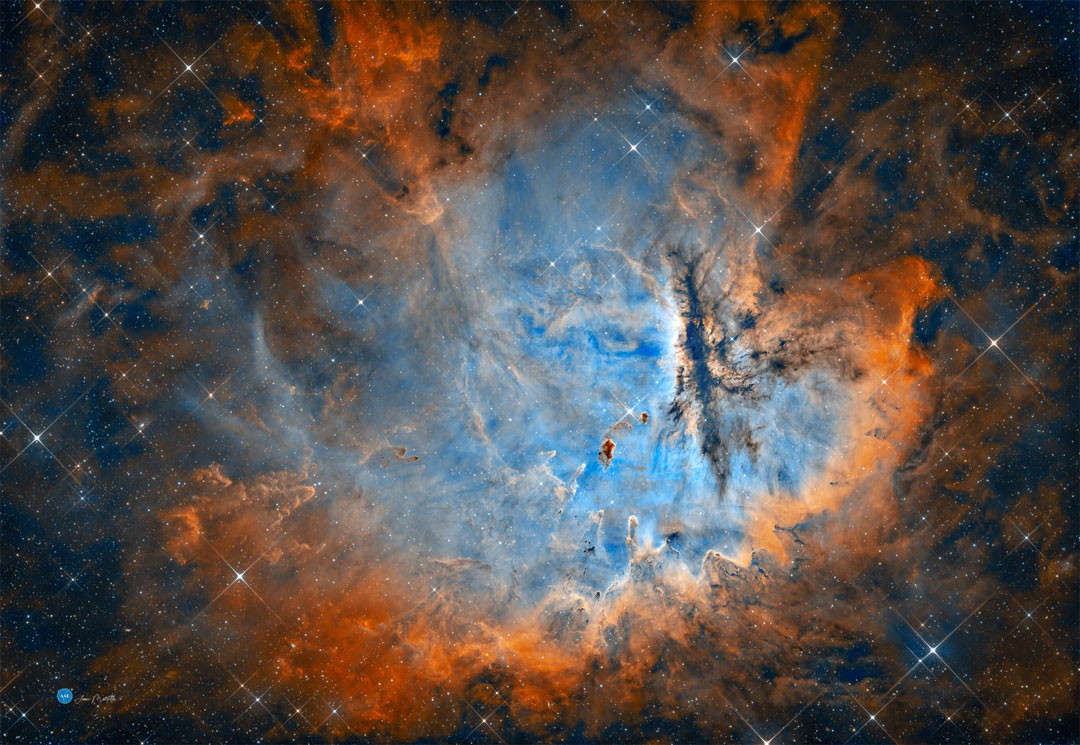
- We can also get multiple images by using both
start_date
andend_date
, or usingcount
.
= 5 nimg
= 'https://api.nasa.gov/planetary/apod' +\
nasa_url '?' +\
'api_key=' +\
+\
API_KEY '&' +\
'count=' +\
str(nimg)
= requests.get(nasa_url).json() nasa_data
pd.DataFrame(nasa_data)
date | explanation | hdurl | media_type | service_version | title | url | |
---|---|---|---|---|---|---|---|
0 | 2006-01-21 | In November of 1969, Apollo 12 astronaut-photo... | https://apod.nasa.gov/apod/image/0601/bean_con... | image | v1 | Apollo 12: Self-Portrait | https://apod.nasa.gov/apod/image/0601/bean_con... |
1 | 1996-08-04 | A bird? A plane? No, but pictured here is some... | https://apod.nasa.gov/apod/image/ngc3393_hst_b... | image | v1 | NGC 3393: A Super Spiral? | https://apod.nasa.gov/apod/image/ngc3393_hst.gif |
2 | 1998-10-17 | This cluster of stars, known as G1, is the bri... | https://apod.nasa.gov/apod/image/9810/m31gc1_h... | image | v1 | A Giant Globular Cluster in M31 | https://apod.nasa.gov/apod/image/9810/m31gc1_h... |
3 | 2021-04-01 | Have you ever seen a rocket launch -- from spa... | NaN | video | v1 | Rocket Launch as Seen from the Space Station | https://www.youtube.com/embed/B1R3dTdcpSU?rel=0 |
4 | 2014-06-08 | Jewels don't shine this bright -- only stars d... | https://apod.nasa.gov/apod/image/1406/ngc290_h... | image | v1 | Open Cluster NGC 290: A Stellar Jewel Box | https://apod.nasa.gov/apod/image/1406/ngc290_h... |
Example: Rutgers Courses
The Rutgers course catalog, for a given subject, term, campus and level (e.g. graduate) are given on the website: https://classes.rutgers.edu/soc/#courses?subject=960&semester=12025&campus=NB&level=G
Inspecting the requests made from the webpage (soc_utils.js
) reveals API requests of the form
https://classes.rutgers.edu/soc/api/courses.json?year=2024&term=9&campus=NB
Parameters:
term=
0
: winter1
: spring7
: summer9
: fall
= requests.get('https://classes.rutgers.edu/soc/api/courses.json?year=2025&term=9&campus=NB')
response response
<Response [200]>
0].keys() response.json()[
dict_keys(['campusLocations', 'subject', 'openSections', 'synopsisUrl', 'title', 'preReqNotes', 'courseString', 'school', 'credits', 'subjectDescription', 'coreCodes', 'expandedTitle', 'courseFeeDescr', 'mainCampus', 'subjectNotes', 'courseNumber', 'creditsObject', 'level', 'campusCode', 'subjectGroupNotes', 'offeringUnitCode', 'offeringUnitTitle', 'courseDescription', 'sections', 'supplementCode', 'courseFee', 'unitNotes', 'courseNotes'])
## Parsing individual fields
0]['mainCampus'] response.json()[
'NB'
0]['sections'][0]['instructorsText'] response.json()[
'HABERL, CHARLES'
0]['sections'][0]['meetingTimes'][0]['meetingDay'] response.json()[
'H'
0]['sections'][0]['meetingTimes'][0]['startTime'] response.json()[
'0200'
0]['sections'][0]['meetingTimes'][0]['endTime'] response.json()[
'0320'
0]['level'] response.json()[
'U'
0]['title'] response.json()[
'BIBLE IN ARAMAIC'
0]['expandedTitle'] response.json()[
'THE BIBLE IN ARAMAIC '
## Constructing a pandas df
= []
data for course in response.json():
for section in course['sections']:
= dict(title=course['expandedTitle'],
d =course['level'],
level=section['instructorsText'],
instructor=course['courseString'],
courseString=section['meetingTimes'][0]['meetingDay'],
meeting1day=section['meetingTimes'][0]['startTime'],
meeting1startTime=section['meetingTimes'][0]['endTime'],
meeting1endTime=course['courseNumber'],
course=course['subject'],
subject=course['school']['code'],
school_code=course['school']['description']
school
)if len(section['meetingTimes']) >= 2:
'meeting2day'] = section['meetingTimes'][1]['meetingDay']
d['meeting2startTime'] = section['meetingTimes'][1]['startTime']
d['meeting2endTime'] = section['meetingTimes'][1]['endTime']
d[
data.append(d)
= pd.DataFrame(data) df
## Filtering using pandas
== '954', :] df.loc[df.subject
title | level | instructor | courseString | meeting1day | meeting1startTime | meeting1endTime | course | subject | school_code | school | meeting2day | meeting2startTime | meeting2endTime | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
10150 | STATISTICAL LEARNING FOR DATA SCIENCE ... | G | 16:954:534 | H | 0600 | 0900 | 534 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN | |
10151 | STATISTICAL LEARNING FOR DATA SCIENCE ... | G | MORAN, GEMMA | 16:954:534 | M | 0350 | 0510 | 534 | 954 | 16 | The School of Graduate Studies - New Brunswick | W | 0350 | 0510 |
10152 | ADVANCED ANALYTICS USING STATISTICAL SOFTWARE ... | G | JOHN, AJITA | 16:954:577 | W | 0600 | 0900 | 577 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
10153 | PROBABILITY AND STATISTICAL INFERENCE FOR DATA... | G | WANG, SIJIAN | 16:954:581 | M | 0600 | 0900 | 581 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
10154 | PROBABILITY AND STATISTICAL INFERENCE FOR DATA... | G | WANG, SIJIAN | 16:954:581 | M | 0600 | 0900 | 581 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
10155 | REGRESSION AND TIME SERIES ANALYSIS FOR DATA S... | G | CHEN, YAQING | 16:954:596 | W | 0830 | 1130 | 596 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
10156 | REGRESSION AND TIME SERIES ANALYSIS FOR DATA S... | G | HU, YIFAN | 16:954:596 | T | 0600 | 0900 | 596 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
10157 | INDEPENDENT STUDIES IN THE APPLICATION OF DATA... | G | 16:954:683 | 683 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN | ||||
10158 | PRACTICAL TRAINING IN STATISTICS FOR DATA SCIE... | G | 16:954:690 | 690 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN | ||||
10159 | SPECIAL TOPICS IN DATA SCIENCE ... | G | ZHENG, JI | 16:954:694 | T | 0600 | 0900 | 694 | 954 | 16 | The School of Graduate Studies - New Brunswick | NaN | NaN | NaN |
## Interactive dataframe (interaction possible in a quarto website)
from itables import show
=0) show(df, maxBytes
title | level | instructor | courseString | meeting1day | meeting1startTime | meeting1endTime | course | subject | school_code | school | meeting2day | meeting2startTime | meeting2endTime |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Loading ITables v2.2.5 from the internet... (need help?) |
Example: Rutgers Bus
Passio GO is a free customer facing app or web browser, offering route, stop, and more information to riders.
Rutgers uses Passio GO to track the school buses.
The Python package passiogo
is an open source project to help get Passio GO data.
Install passiogo
in your conda environment:
pip install passiogo
We first use the passiogo
package to get Rutgers bus route and stop IDs.
import passiogo
= passiogo.getSystemFromID(1268) system
= system.getRoutes() routes
0].__dict__ routes[
{'id': '22103',
'groupId': None,
'groupColor': None,
'name': 'Camden',
'shortName': 'Cam',
'nameOrig': 'Camden',
'fullname': 'Rutgers University',
'myid': '41231',
'mapApp': '1',
'archive': '0',
'goPrefixRouteName': '1',
'goShowSchedule': 0,
'outdated': '0',
'distance': 670,
'latitude': '39.952097000',
'longitude': '-75.126481000',
'serviceTime': None,
'serviceTimeShort': None,
'systemId': 1268,
'system': <passiogo.TransportationSystem at 0x147bd6480>}
= []
routes_list for i in range(len(routes)):
routes_list.append(routes[i].__dict__)
= pd.DataFrame(routes_list)
routes_df routes_df.head()
id | groupId | groupColor | name | shortName | nameOrig | fullname | myid | mapApp | archive | goPrefixRouteName | goShowSchedule | outdated | distance | latitude | longitude | serviceTime | serviceTimeShort | systemId | system | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 22103 | None | None | Camden | Cam | Camden | Rutgers University | 41231 | 1 | 0 | 1 | 0 | 0 | 670 | 39.952097000 | -75.126481000 | None | None | 1268 | <passiogo.TransportationSystem object at 0x147... |
1 | 10052 | None | None | H Route | H | H Route | Rutgers University | 55368 | 1 | 0 | 1 | 0 | 0 | 721 | 40.523795537 | -74.458255043 | None | None | 1268 | <passiogo.TransportationSystem object at 0x147... |
2 | 10060 | None | None | C Route | C | C Route | Rutgers University | 55366 | 1 | 0 | 1 | 0 | 0 | 721 | 40.514556942 | -74.466148593 | None | None | 1268 | <passiogo.TransportationSystem object at 0x147... |
3 | 10037 | None | None | REXL Route | REXL | REXL Route | Rutgers University | 54551 | 1 | 0 | 1 | 0 | 0 | 721 | 40.482986940 | -74.437534636 | None | None | 1268 | <passiogo.TransportationSystem object at 0x147... |
4 | 10035 | None | None | WKND1 Weekend 1 | WKND1 | Weekend 1 | Rutgers University | 26435 | 1 | 0 | 1 | 0 | 1 | 721 | 40.503664735 | -74.452402128 | is not provided: no bus on the route | No bus in service | 1268 | <passiogo.TransportationSystem object at 0x147... |
= system.getStops() stops
= []
stops_list for i in range(len(stops)):
stops_list.append(stops[i].__dict__)
= pd.DataFrame(stops_list)
stops_df stops_df.head()
id | routesAndPositions | systemId | name | latitude | longitude | radius | system | |
---|---|---|---|---|---|---|---|---|
0 | 10098 | {'4056': [0], '4098': [0]} | 1268 | Newark Penn Station | 40.734819 | -74.164721 | 50 | <passiogo.TransportationSystem object at 0x147... |
1 | 10053 | {'4056': [1], '4063': [4], '4088': [1], '4098'... | 1268 | Medical School | 40.739617 | -74.189267 | 50 | <passiogo.TransportationSystem object at 0x147... |
2 | 10066 | {'4056': [2], '4098': [2]} | 1268 | Hospital | 40.741868 | -74.191581 | 40 | <passiogo.TransportationSystem object at 0x147... |
3 | 10057 | {'4056': [3]} | 1268 | Bergen Bldg (back) | 40.743466 | -74.192475 | 40 | <passiogo.TransportationSystem object at 0x147... |
4 | 10079 | {'4056': [4]} | 1268 | Dental School | 40.742285 | -74.190294 | 40 | <passiogo.TransportationSystem object at 0x147... |
'latitude'] = pd.to_numeric(stops_df['latitude'])
stops_df['longitude'] = pd.to_numeric(stops_df['longitude']) stops_df[
import plotly.express as px
='latitude', lon='longitude') px.scatter_map(stops_df, lat
= stops_df[stops_df.name.str.lower().str.contains('hill')]
hill_stops hill_stops
id | routesAndPositions | systemId | name | latitude | longitude | radius | system | |
---|---|---|---|---|---|---|---|---|
20 | 10034 | {'26435': [3], '37199': [3], '46583': [3], '54... | 1268 | Hill Center (NB) | 40.521919 | -74.463298 | 50 | <passiogo.TransportationSystem object at 0x147... |
36 | 21050 | {'26436': [15], '46584': [15], '54550': [5], '... | 1268 | Hill Center (SB) | 40.521925 | -74.463308 | 50 | <passiogo.TransportationSystem object at 0x147... |
ETAs
The API format for obtaining bus ETAs is:
https://passiogo.com/mapGetData.php?eta=3&deviceId={random.randint(10000000,99999999)}&stopIds={id}
(Note: the URL above is is from here).
= hill_stops['id'].iloc[1] stop_id
= f'https://passiogo.com/mapGetData.php?eta=3&deviceId={np.random.randint(10000000,99999999)}&stopIds={stop_id}' url
= requests.get(url) req
= []
hill_etas for bus in req.json()['ETAs']['21050']:
'route': bus['theStop']['routeName'],
hill_etas.append({'eta': bus['eta']})
pd.DataFrame(hill_etas)
route | eta | |
---|---|---|
0 | H Route | arrived |
1 | REXB Route | arriving |
2 | C Route | less than 1 min |
Note: A developer named Piero Maddaleni has made Transitstatus, an open source project tracking transit using PassioGO data. Check it out here!
Example: Film permits in NYC
NYC (and many other cities!) has many public datasets. Let’s look at film permits in NYC.
If you click on “Actions” then “API”, you see:
NYC Open Data uses the Socrata Open Data API. The Socrata Open Data API allows you to programmatically access a wealth of open data resources from governments, non-profits, and NGOs around the world.
Here is the documentation specifically for the film permits data, which let’s us know what parameters are available.
= 'https://data.cityofnewyork.us/resource/tg4x-b46p.json'
json_url
= requests.get(json_url)
req req
<Response [200]>
300] req.content[:
b'[{"eventid":"831933","eventtype":"Shooting Permit","startdatetime":"2025-01-24T07:00:00.000","enddatetime":"2025-01-24T21:00:00.000","enteredon":"2025-01-21T16:44:25.000","eventagency":"Mayor\'s Office of Media & Entertainment","parkingheld":"KINGSLAND AVENUE between GREENPOINT AVENUE and NORMAN AVEN'
0] req.json()[
{'eventid': '831933',
'eventtype': 'Shooting Permit',
'startdatetime': '2025-01-24T07:00:00.000',
'enddatetime': '2025-01-24T21:00:00.000',
'enteredon': '2025-01-21T16:44:25.000',
'eventagency': "Mayor's Office of Media & Entertainment",
'parkingheld': 'KINGSLAND AVENUE between GREENPOINT AVENUE and NORMAN AVENUE, MONITOR STREET between GREENPOINT AVENUE and NORMAN AVENUE, MORGAN AVENUE between NASSAU AVENUE and DRIGGS AVENUE, NASSAU AVENUE between SUTTON STREET and HAUSMAN STREET, NASSAU AVENUE between HAUSMAN STREET and APOLLO STREET, MORGAN AVENUE between NASSAU AVENUE and NORMAN AVENUE, SUTTON STREET between NORMAN AVENUE and NASSAU AVENUE, NASSAU AVENUE between SUTTON STREET and KINGSLAND AVENUE',
'borough': 'Brooklyn',
'communityboard_s': '1',
'policeprecinct_s': '94',
'category': 'Television',
'subcategoryname': 'Episodic series',
'country': 'United States of America',
'zipcode_s': '11222'}
= pd.DataFrame(req.json())
df df.head()
eventid | eventtype | startdatetime | enddatetime | enteredon | eventagency | parkingheld | borough | communityboard_s | policeprecinct_s | category | subcategoryname | country | zipcode_s | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 831933 | Shooting Permit | 2025-01-24T07:00:00.000 | 2025-01-24T21:00:00.000 | 2025-01-21T16:44:25.000 | Mayor's Office of Media & Entertainment | KINGSLAND AVENUE between GREENPOINT AVENUE and... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11222 |
1 | 831853 | Shooting Permit | 2025-01-23T07:00:00.000 | 2025-01-23T21:00:00.000 | 2025-01-21T12:02:30.000 | Mayor's Office of Media & Entertainment | BEDFORD AVENUE between NORTH 4 STREET and N... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11211, 11222, 11249 |
2 | 831842 | Shooting Permit | 2025-01-24T07:00:00.000 | 2025-01-24T23:00:00.000 | 2025-01-21T11:19:18.000 | Mayor's Office of Media & Entertainment | 30 STREET between 47 AVENUE and HUNTERS POINT ... | Queens | 2 | 108 | Television | Cable-episodic | United States of America | 11101 |
3 | 831840 | Shooting Permit | 2025-01-24T06:30:00.000 | 2025-01-24T21:00:00.000 | 2025-01-21T11:10:17.000 | Mayor's Office of Media & Entertainment | CALYER STREET between DIAMOND STREET and JEWEL... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11222 |
4 | 831802 | Shooting Permit | 2025-01-22T07:00:00.000 | 2025-01-22T21:00:00.000 | 2025-01-21T09:15:26.000 | Mayor's Office of Media & Entertainment | KINGLAND AVENUE between GREENPOINT AVENUE and ... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11222 |
We can also change the URL to filter the data:
= 'https://data.cityofnewyork.us/resource/tg4x-b46p.json?borough=Manhattan'
url = requests.get(url)
req pd.DataFrame(req.json()).head()
eventid | eventtype | startdatetime | enddatetime | enteredon | eventagency | parkingheld | borough | communityboard_s | policeprecinct_s | category | subcategoryname | country | zipcode_s | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 753784 | Theater Load in and Load Outs | 2023-12-24T00:01:00.000 | 2023-12-31T23:59:00.000 | 2023-12-18T12:59:21.000 | Mayor's Office of Media & Entertainment | WEST 62 STREET between COLUMBUS AVENUE and A... | Manhattan | 7 | 20 | Theater | Theater | United States of America | 10023 |
1 | 752706 | Shooting Permit | 2023-12-13T06:00:00.000 | 2023-12-13T20:00:00.000 | 2023-12-06T09:01:38.000 | Mayor's Office of Media & Entertainment | HUDSON STREET between MORTON STREET and BARROW... | Manhattan | 1, 2, 3 | 5, 6, 7, 84, 90 | Film | Feature | United States of America | 10002, 10014, 10038, 11201, 11211 |
2 | 752753 | Theater Load in and Load Outs | 2023-12-12T01:00:00.000 | 2023-12-13T01:00:00.000 | 2023-12-06T14:10:53.000 | Mayor's Office of Media & Entertainment | WEST 16 STREET between 9 AVENUE and 10 AVENUE | Manhattan | 4 | 10 | Theater | Theater | United States of America | 10011 |
3 | 752512 | Shooting Permit | 2023-12-12T07:00:00.000 | 2023-12-12T23:59:00.000 | 2023-12-04T15:01:57.000 | Mayor's Office of Media & Entertainment | ST NICHOLAS PLACE between WEST 155 STREET and... | Manhattan | 10, 12, 9 | 30, 32, 33 | Television | Episodic series | United States of America | 10031, 10032, 10039 |
4 | 752438 | Shooting Permit | 2023-12-15T01:00:00.000 | 2023-12-15T11:00:00.000 | 2023-12-04T08:02:57.000 | Mayor's Office of Media & Entertainment | WEST 47 STREET between 6 AVENUE and 7 AVENUE | Manhattan | 5 | 18 | Television | News | United States of America | 10036 |
For the Socrata Open Data API, we can also use “Socrata Query Language” to select our data.
For example, if we want to select more than the default 1000 rows, we can use $limit=2000
.
= 'https://data.cityofnewyork.us/resource/tg4x-b46p.json?$limit=2000'
url = requests.get(url)
req = pd.DataFrame(req.json())
film_df film_df.shape
(2000, 14)
0:2, :] film_df.iloc[
eventid | eventtype | startdatetime | enddatetime | enteredon | eventagency | parkingheld | borough | communityboard_s | policeprecinct_s | category | subcategoryname | country | zipcode_s | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 831933 | Shooting Permit | 2025-01-24T07:00:00.000 | 2025-01-24T21:00:00.000 | 2025-01-21T16:44:25.000 | Mayor's Office of Media & Entertainment | KINGSLAND AVENUE between GREENPOINT AVENUE and... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11222 |
1 | 831853 | Shooting Permit | 2025-01-23T07:00:00.000 | 2025-01-23T21:00:00.000 | 2025-01-21T12:02:30.000 | Mayor's Office of Media & Entertainment | BEDFORD AVENUE between NORTH 4 STREET and N... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11211, 11222, 11249 |
We can also use $offset
to start data collection after a certain number of rows.
= 'https://data.cityofnewyork.us/resource/tg4x-b46p.json?$limit=2000&$offset=1'
url = requests.get(url)
req 0:2, :] pd.DataFrame(req.json()).iloc[
eventid | eventtype | startdatetime | enddatetime | enteredon | eventagency | parkingheld | borough | communityboard_s | policeprecinct_s | category | subcategoryname | country | zipcode_s | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 831853 | Shooting Permit | 2025-01-23T07:00:00.000 | 2025-01-23T21:00:00.000 | 2025-01-21T12:02:30.000 | Mayor's Office of Media & Entertainment | BEDFORD AVENUE between NORTH 4 STREET and N... | Brooklyn | 1 | 94 | Television | Episodic series | United States of America | 11211, 11222, 11249 |
1 | 831842 | Shooting Permit | 2025-01-24T07:00:00.000 | 2025-01-24T23:00:00.000 | 2025-01-21T11:19:18.000 | Mayor's Office of Media & Entertainment | 30 STREET between 47 AVENUE and HUNTERS POINT ... | Queens | 2 | 108 | Television | Cable-episodic | United States of America | 11101 |
We won’t do any further analysis of this data, but here is a great analysis of this dataset if you are interested!
Example: CDC COVID Cases
The CDC also uses the Socrata Open Data API.
Let’s look at these COVID cases data.
The API url is: https://data.cdc.gov/resource/n8mc-b4w4.json
From the documentation, we see there are a number of parameters. We consider:
res_state=NY
case_month=2021-09
= 'https://data.cdc.gov/resource/n8mc-b4w4.json?res_state=NY&case_month=2021-09'
url = requests.get(url)
req pd.DataFrame(req.json()).tail()
case_month | res_state | state_fips_code | res_county | county_fips_code | age_group | sex | race | ethnicity | process | exposure_yn | current_status | symptom_status | hosp_yn | icu_yn | death_yn | case_onset_interval | case_positive_specimen | underlying_conditions_yn | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
995 | 2021-09 | NY | 36 | CORTLAND | 36023 | 18 to 49 years | Male | White | Non-Hispanic/Latino | Missing | Missing | Laboratory-confirmed case | Symptomatic | Missing | Missing | No | 0.0 | NaN | NaN |
996 | 2021-09 | NY | 36 | FRANKLIN | 36033 | 18 to 49 years | Male | NA | NA | Missing | Missing | Laboratory-confirmed case | Missing | Missing | Missing | No | NaN | NaN | NaN |
997 | 2021-09 | NY | 36 | ALLEGANY | 36003 | 65+ years | Female | White | Non-Hispanic/Latino | Missing | Missing | Laboratory-confirmed case | Symptomatic | No | Missing | No | 0.0 | NaN | NaN |
998 | 2021-09 | NY | 36 | TOMPKINS | 36109 | 18 to 49 years | Male | NA | NA | Missing | Missing | Laboratory-confirmed case | Missing | Missing | Missing | No | NaN | NaN | NaN |
999 | 2021-09 | NY | 36 | WARREN | 36113 | 50 to 64 years | Male | Unknown | Unknown | Missing | Missing | Laboratory-confirmed case | Symptomatic | Missing | Missing | No | 0.0 | NaN | NaN |
Example: Open Movie Data Base
OMDB is a web service to obtain movie information. All content and images on the site are contributed and maintained by users.
- Obtain an API key.
- Save API key in `omdb_api.txt’
- Specify URL:
with open('omdb_api.txt', 'r') as file:
= file.read().strip() API_KEY
= 'tt3896198' movie_id
= 'http://www.omdbapi.com/?' +\
omdb_url 'apikey=' +\
+\
API_KEY '&' +\
'i=' +\
movie_id
= requests.get(omdb_url) omdb_data
omdb_data.json()
{'Title': 'Guardians of the Galaxy Vol. 2',
'Year': '2017',
'Rated': 'PG-13',
'Released': '05 May 2017',
'Runtime': '136 min',
'Genre': 'Action, Adventure, Comedy',
'Director': 'James Gunn',
'Writer': 'James Gunn, Dan Abnett, Andy Lanning',
'Actors': 'Chris Pratt, Zoe Saldaña, Dave Bautista',
'Plot': "The Guardians struggle to keep together as a team while dealing with their personal family issues, notably Star-Lord's encounter with his father, the ambitious celestial being Ego.",
'Language': 'English',
'Country': 'United States',
'Awards': 'Nominated for 1 Oscar. 15 wins & 60 nominations total',
'Poster': 'https://m.media-amazon.com/images/M/MV5BNWE5MGI3MDctMmU5Ni00YzI2LWEzMTQtZGIyZDA5MzQzNDBhXkEyXkFqcGc@._V1_SX300.jpg',
'Ratings': [{'Source': 'Internet Movie Database', 'Value': '7.6/10'},
{'Source': 'Rotten Tomatoes', 'Value': '85%'},
{'Source': 'Metacritic', 'Value': '67/100'}],
'Metascore': '67',
'imdbRating': '7.6',
'imdbVotes': '792,363',
'imdbID': 'tt3896198',
'Type': 'movie',
'DVD': 'N/A',
'BoxOffice': '$389,813,101',
'Production': 'N/A',
'Website': 'N/A',
'Response': 'True'}
Example: Spoonacular
Spoonacular is a food and recipe API.
- Sign up for an API key here.
There are different URL formats for different tasks.
Obtaining Recipe Information
Documentation here.
URL format:
https://api.spoonacular.com/recipes/{recipe-id}/information?apiKey=YOUR-API-KEY
URL format with nutrition information:
https://api.spoonacular.com/recipes/{recipe-id}/information?apiKey=YOUR-API-KEY&includeNutrition=true
with open('spoon_api.txt', 'r') as file:
= file.read().strip() API_KEY
= str(716429)
recipe_id = 'https://api.spoonacular.com/recipes/' +\
spoon_url +\
recipe_id '/information?' +\
'apiKey=' +\
+\
API_KEY '&includeNutrition=true'
= requests.get(spoon_url) spoon_data
spoon_data.json().keys()
dict_keys(['id', 'image', 'imageType', 'title', 'readyInMinutes', 'servings', 'sourceUrl', 'vegetarian', 'vegan', 'glutenFree', 'dairyFree', 'veryHealthy', 'cheap', 'veryPopular', 'sustainable', 'lowFodmap', 'weightWatcherSmartPoints', 'gaps', 'preparationMinutes', 'cookingMinutes', 'aggregateLikes', 'healthScore', 'creditsText', 'license', 'sourceName', 'pricePerServing', 'extendedIngredients', 'nutrition', 'summary', 'cuisines', 'dishTypes', 'diets', 'occasions', 'instructions', 'analyzedInstructions', 'originalId', 'spoonacularScore', 'spoonacularSourceUrl'])
'nutrition']['nutrients'][0] spoon_data.json()[
{'name': 'Calories',
'amount': 543.36,
'unit': 'kcal',
'percentOfDailyNeeds': 27.17}
Search for Recipes
We use the parameters:
cuisine=Mexican
intolerances=Dairy
= 'https://api.spoonacular.com/recipes/complexSearch?' +\
spoon_url 'apiKey=' +\
+\
API_KEY '&cuisine=Mexican' +\
'&intolerances=Dairy'
= requests.get(spoon_url) spoon_data
spoon_data.json().keys()
dict_keys(['results', 'offset', 'number', 'totalResults'])
'results'][0] spoon_data.json()[
{'id': 640062,
'title': 'Corn Avocado Salsa',
'image': 'https://img.spoonacular.com/recipes/640062-312x231.jpg',
'imageType': 'jpg'}
'results']) pd.DataFrame(spoon_data.json()[
id | title | image | imageType | |
---|---|---|---|---|
0 | 640062 | Corn Avocado Salsa | https://img.spoonacular.com/recipes/640062-312... | jpg |
1 | 715543 | Homemade Guacamole | https://img.spoonacular.com/recipes/715543-312... | jpg |
2 | 715391 | Slow Cooker Chicken Taco Soup | https://img.spoonacular.com/recipes/715391-312... | jpg |
3 | 982382 | Instant Pot Chicken Taco Soup | https://img.spoonacular.com/recipes/982382-312... | jpg |
4 | 637157 | Carolina Caviar - Black Bean Salsa | https://img.spoonacular.com/recipes/637157-312... | jpg |
5 | 664501 | Vegan Taco bowls with Cilantro Lime Cauliflowe... | https://img.spoonacular.com/recipes/664501-312... | jpg |
6 | 645856 | Grilled Salmon With Cherry, Pineapple, Mango S... | https://img.spoonacular.com/recipes/645856-312... | jpg |
7 | 716290 | Mango Salsa | https://img.spoonacular.com/recipes/716290-312... | jpg |
8 | 638588 | Chilled Avocado and Cucumber Soup With Prawn a... | https://img.spoonacular.com/recipes/638588-312... | jpg |
9 | 643061 | Flank Steak with Herbed Salsa | https://img.spoonacular.com/recipes/643061-312... | jpg |
Wine recommendations
We use the parameters:
wine=merlot
number=5
= 'https://api.spoonacular.com/food/wine/recommendation?' +\
spoon_url 'apiKey=' +\
+\
API_KEY '&wine=merlot' +\
'&number=5'
= requests.get(spoon_url) spoon_wine
'recommendedWines']) pd.DataFrame(spoon_wine.json()[
id | title | description | price | imageUrl | averageRating | ratingCount | score | link | |
---|---|---|---|---|---|---|---|---|---|
0 | 428396 | Rolling Stones 50th Anniversary Forty Licks Me... | The 2012 Merlot captures the attitude of Mendo... | $16.99 | https://img.spoonacular.com/products/428396-31... | 0.96 | 7.0 | 0.9145 | https://www.amazon.com/Rolling-Stones-Annivers... |
1 | 431508 | Madsen Family Cellars State Merlot Wine | This well-structured blend of Red Mountain and... | $22.0 | https://img.spoonacular.com/products/431508-31... | 1.00 | 3.0 | 0.9000 | https://www.amazon.com/Madsen-Family-Cellars-W... |
2 | 428495 | Columbia Winery Merlot | The deep purple color of our Columbia Merlot a... | $22.65 | https://img.spoonacular.com/products/428495-31... | 1.00 | 3.0 | 0.9000 | https://www.amazon.com/Columbia-Winery-Valley-... |
3 | 435229 | Chateau Ste. Michelle Indian Wells Vineyard Me... | Ripe and luscious black cherry and berry aroma... | $16.99 | https://img.spoonacular.com/products/435229-31... | 0.94 | 6.0 | 0.8874 | https://click.linksynergy.com/deeplink?id=*QCi... |
4 | 436263 | Edna Valley Vineyard Merlot | Edna Valley Vineyard 2010 Merlot opens with ar... | $12.99 | https://img.spoonacular.com/products/436263-31... | 0.94 | 5.0 | 0.8775 | https://click.linksynergy.com/deeplink?id=*QCi... |
Example: Federal Reserve Economic Data
The St Louis Federal Reserve Bank has an API to access Federal Reserve Economic Data.
Searching for series
The API to search for available data series:
https://api.stlouisfed.org/fred/series/search
For example, we can get the id
of economic series matching a search query - here we consider monetary+service+index
, following the documentation.
with open('fed_api.txt', 'r') as file:
= file.read().strip() API_KEY
= f'https://api.stlouisfed.org/fred/series/search?search_text=monetary+service+index&api_key={API_KEY}&file_type=json' url
= requests.get(url) req
req.json().keys()
dict_keys(['realtime_start', 'realtime_end', 'order_by', 'sort_order', 'count', 'offset', 'limit', 'seriess'])
'seriess']).head() pd.DataFrame(req.json()[
id | realtime_start | realtime_end | title | observation_start | observation_end | frequency | frequency_short | units | units_short | seasonal_adjustment | seasonal_adjustment_short | last_updated | popularity | group_popularity | notes | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | MSIM2 | 2025-04-24 | 2025-04-24 | Monetary Services Index: M2 (preferred) | 1967-01-01 | 2013-12-01 | Monthly | M | Billions of Dollars | Bil. of $ | Seasonally Adjusted | SA | 2014-01-17 07:16:44-06 | 19 | 19 | The MSI measure the flow of monetary services ... |
1 | MSIMZMP | 2025-04-24 | 2025-04-24 | Monetary Services Index: MZM (preferred) | 1967-01-01 | 2013-12-01 | Monthly | M | Billions of Dollars | Bil. of $ | Seasonally Adjusted | SA | 2014-01-17 07:16:42-06 | 14 | 14 | The MSI measure the flow of monetary services ... |
2 | MSIALLP | 2025-04-24 | 2025-04-24 | Monetary Services Index: ALL Assets (preferred) | 1967-01-01 | 2013-12-01 | Monthly | M | Billions of Dollars | Bil. of $ | Seasonally Adjusted | SA | 2014-01-17 07:16:45-06 | 10 | 10 | The MSI measure the flow of monetary services ... |
3 | OCM2P | 2025-04-24 | 2025-04-24 | Real User Cost Index of MSI-M2 (preferred) | 1967-01-01 | 2013-12-01 | Monthly | M | Percent | % | Not Seasonally Adjusted | NSA | 2014-01-17 07:16:41-06 | 6 | 6 | Preferred benchmark rate equals 100 basis poin... |
4 | MSIMZMA | 2025-04-24 | 2025-04-24 | Monetary Services Index: MZM (alternative) | 1967-01-01 | 2013-12-01 | Monthly | M | Billions of Dollars | Bil. of $ | Seasonally Adjusted | SA | 2014-01-17 07:16:43-06 | 4 | 4 | The MSI measure the flow of monetary services ... |
Obtaining observations
Once we have a series id
, we can use it to obtain the actual observations.
For this we use the API:
https://api.stlouisfed.org/fred/series/observations
Here is the documentation with parameter details.
Let’s get the SP500 data using series_id=SP500
= f'https://api.stlouisfed.org/fred/series/observations?series_id=SP500&api_key={API_KEY}&file_type=json'
url_sp500 = requests.get(url_sp500) req
= list(req.json().keys())
keys keys
['realtime_start',
'realtime_end',
'observation_start',
'observation_end',
'units',
'output_type',
'file_type',
'order_by',
'sort_order',
'count',
'offset',
'limit',
'observations']
'observations']).head() pd.DataFrame(req.json()[
realtime_start | realtime_end | date | value | |
---|---|---|---|---|
0 | 2025-04-23 | 2025-04-23 | 2015-04-24 | 2117.69 |
1 | 2025-04-23 | 2025-04-23 | 2015-04-27 | 2108.92 |
2 | 2025-04-23 | 2025-04-23 | 2015-04-28 | 2114.76 |
3 | 2025-04-23 | 2025-04-23 | 2015-04-29 | 2106.85 |
4 | 2025-04-23 | 2025-04-23 | 2015-04-30 | 2085.51 |
Example: World Bank API
The World Bank provides an API to retrieve up-to-date economic and health indicators from countries worldwide.
An excel spreadsheet of all indicators is given here.
- Retrieving the population indicator
= 'http://api.worldbank.org/v2/country/all/indicator/SP.POP.TOTL?format=json'
url_json_population = requests.get(url_json_population) req
1][0] req.json()[
{'indicator': {'id': 'SP.POP.TOTL', 'value': 'Population, total'},
'country': {'id': 'ZH', 'value': 'Africa Eastern and Southern'},
'countryiso3code': 'AFE',
'date': '2024',
'value': None,
'unit': '',
'obs_status': '',
'decimal': 0}
1]).head(3) pd.DataFrame(req.json()[
indicator | country | countryiso3code | date | value | unit | obs_status | decimal | |
---|---|---|---|---|---|---|---|---|
0 | {'id': 'SP.POP.TOTL', 'value': 'Population, to... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2024 | NaN | 0 | ||
1 | {'id': 'SP.POP.TOTL', 'value': 'Population, to... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2023 | 750503764.0 | 0 | ||
2 | {'id': 'SP.POP.TOTL', 'value': 'Population, to... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2022 | 731821393.0 | 0 |
- Retrieving infant deaths indicator
= 'http://api.worldbank.org/v2/country/all/indicator/SH.DTH.IMRT?format=json'
url_json_infant_deaths = requests.get(url_json_infant_deaths) req2
1]).head(3) pd.DataFrame(req2.json()[
indicator | country | countryiso3code | date | value | unit | obs_status | decimal | |
---|---|---|---|---|---|---|---|---|
0 | {'id': 'SH.DTH.IMRT', 'value': 'Number of infa... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2024 | NaN | 0 | ||
1 | {'id': 'SH.DTH.IMRT', 'value': 'Number of infa... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2023 | 924427.0 | 0 | ||
2 | {'id': 'SH.DTH.IMRT', 'value': 'Number of infa... | {'id': 'ZH', 'value': 'Africa Eastern and Sout... | AFE | 2022 | 953019.0 | 0 |
Package wbgapi
The wbgapi
python package (pip install wbgapi
) provides an easy access to data from the World Bank, without needing to perform the HTTP requests ourselves.
import wbgapi as wb
Let’s see what kind of information we have available.
for i, indicator in enumerate(wb.economy.list()):
print(indicator)
if i > 5: break
{'id': 'ABW', 'value': 'Aruba', 'aggregate': False, 'longitude': -70.0167, 'latitude': 12.5167, 'region': 'LCN', 'adminregion': '', 'lendingType': 'LNX', 'incomeLevel': 'HIC', 'capitalCity': 'Oranjestad'}
{'id': 'AFE', 'value': 'Africa Eastern and Southern', 'aggregate': True, 'longitude': None, 'latitude': None, 'region': '', 'adminregion': '', 'lendingType': '', 'incomeLevel': '', 'capitalCity': ''}
{'id': 'AFG', 'value': 'Afghanistan', 'aggregate': False, 'longitude': 69.1761, 'latitude': 34.5228, 'region': 'SAS', 'adminregion': 'SAS', 'lendingType': 'IDX', 'incomeLevel': 'LIC', 'capitalCity': 'Kabul'}
{'id': 'AFW', 'value': 'Africa Western and Central', 'aggregate': True, 'longitude': None, 'latitude': None, 'region': '', 'adminregion': '', 'lendingType': '', 'incomeLevel': '', 'capitalCity': ''}
{'id': 'AGO', 'value': 'Angola', 'aggregate': False, 'longitude': 13.242, 'latitude': -8.81155, 'region': 'SSF', 'adminregion': 'SSA', 'lendingType': 'IBD', 'incomeLevel': 'LMC', 'capitalCity': 'Luanda'}
{'id': 'ALB', 'value': 'Albania', 'aggregate': False, 'longitude': 19.8172, 'latitude': 41.3317, 'region': 'ECS', 'adminregion': 'ECA', 'lendingType': 'IBD', 'incomeLevel': 'UMC', 'capitalCity': 'Tirane'}
{'id': 'AND', 'value': 'Andorra', 'aggregate': False, 'longitude': 1.5218, 'latitude': 42.5075, 'region': 'ECS', 'adminregion': '', 'lendingType': 'LNX', 'incomeLevel': 'HIC', 'capitalCity': 'Andorra la Vella'}
wb.economy.DataFrame().head()
name | aggregate | longitude | latitude | region | adminregion | lendingType | incomeLevel | capitalCity | |
---|---|---|---|---|---|---|---|---|---|
id | |||||||||
ABW | Aruba | False | -70.0167 | 12.51670 | LCN | LNX | HIC | Oranjestad | |
AFE | Africa Eastern and Southern | True | NaN | NaN | |||||
AFG | Afghanistan | False | 69.1761 | 34.52280 | SAS | SAS | IDX | LIC | Kabul |
AFW | Africa Western and Central | True | NaN | NaN | |||||
AGO | Angola | False | 13.2420 | -8.81155 | SSF | SSA | IBD | LMC | Luanda |
for i, indicator in enumerate(wb.region.list()):
print(indicator)
if i > 5: break
{'id': '', 'code': 'AFE', 'iso2code': 'ZH', 'name': 'Africa Eastern and Southern'}
{'id': '', 'code': 'AFR', 'iso2code': 'A9', 'name': 'Africa'}
{'id': '', 'code': 'AFW', 'iso2code': 'ZI', 'name': 'Africa Western and Central'}
{'id': '', 'code': 'ARB', 'iso2code': '1A', 'name': 'Arab World'}
{'id': '', 'code': 'CAA', 'iso2code': 'C9', 'name': 'Sub-Saharan Africa (IFC classification)'}
{'id': '', 'code': 'CEA', 'iso2code': 'C4', 'name': 'East Asia and the Pacific (IFC classification)'}
{'id': '', 'code': 'CEB', 'iso2code': 'B8', 'name': 'Central Europe and the Baltics'}
for i, indicator in enumerate(wb.series.list()):
print(indicator)
if i > 5: break
{'id': 'AG.CON.FERT.PT.ZS', 'value': 'Fertilizer consumption (% of fertilizer production)'}
{'id': 'AG.CON.FERT.ZS', 'value': 'Fertilizer consumption (kilograms per hectare of arable land)'}
{'id': 'AG.LND.AGRI.K2', 'value': 'Agricultural land (sq. km)'}
{'id': 'AG.LND.AGRI.ZS', 'value': 'Agricultural land (% of land area)'}
{'id': 'AG.LND.ARBL.HA', 'value': 'Arable land (hectares)'}
{'id': 'AG.LND.ARBL.HA.PC', 'value': 'Arable land (hectares per person)'}
{'id': 'AG.LND.ARBL.ZS', 'value': 'Arable land (% of land area)'}
'SH.DTH.IMRT', 'NY.GDP.PCAP.CD']) wb.series.info([
id | value |
---|---|
NY.GDP.PCAP.CD | GDP per capita (current US$) |
SH.DTH.IMRT | Number of infant deaths |
2 elements |
'CAN', 'MEX', 'SEN', 'IND']) # Countries in North America wb.economy.info([
id | value | region | incomeLevel |
---|---|---|---|
CAN | Canada | NAC | HIC |
IND | India | SAS | LMC |
MEX | Mexico | LCN | UMC |
SEN | Senegal | SSF | LMC |
4 elements |
Retrieving specific indicators:
# population for African countries, every other year
= wb.data.DataFrame('SP.POP.TOTL',
df_population 'AFR'),
wb.region.members(range(2010, 2020))
df_population.head()
YR2010 | YR2011 | YR2012 | YR2013 | YR2014 | YR2015 | YR2016 | YR2017 | YR2018 | YR2019 | |
---|---|---|---|---|---|---|---|---|---|---|
economy | ||||||||||
AGO | 23294825.0 | 24218352.0 | 25177394.0 | 26165620.0 | 27160769.0 | 28157798.0 | 29183070.0 | 30234839.0 | 31297155.0 | 32375632.0 |
BDI | 9376444.0 | 9717978.0 | 10071028.0 | 10439341.0 | 10799785.0 | 11047580.0 | 11239451.0 | 11506762.0 | 11859446.0 | 12255336.0 |
BEN | 9797484.0 | 10093623.0 | 10397657.0 | 10708834.0 | 11030004.0 | 11360681.0 | 11697842.0 | 12039780.0 | 12383347.0 | 12726755.0 |
BFA | 16176498.0 | 16661908.0 | 17172287.0 | 17695409.0 | 18229461.0 | 18777487.0 | 19334856.0 | 19894407.0 | 20438288.0 | 20961952.0 |
BWA | 2033111.0 | 2073535.0 | 2108617.0 | 2140682.0 | 2172044.0 | 2203273.0 | 2234776.0 | 2266747.0 | 2299141.0 | 2332083.0 |
Example: arXiv preprint API
The arXiv is a document submission and retrieval system that is heavily used by the physics, mathematics, statistics and computer science communities. It has become the primary means of communicating cutting-edge manuscripts on current and ongoing research. The open-access arXiv e-print repository is available worldwide, and presents no entry barriers to readers. Manuscripts are often submitted to the arXiv before they are published by more traditional means.
API call
The arXiv API calls are made via an HTTP GET or POST requests to an appropriate url. For example, the url
http://export.arxiv.org/api/query?search_query=all:LLM
retrieves results that match the search query all:LLM
.
API data format
Results from the API call are in the Atom 1.0 format.
Atom 1.0 is an xml-based format that is commonly used in website syndication feeds. It is lightweight, and human readable, and results can be cleanly read in many web browsers. For detailed information on Atom, you can read the official Atom 1.0 specification.
Here is a brief, single-entry Atom Feed Document:
<?xml version="1.0" encoding="utf-8"?>
feed xmlns="http://www.w3.org/2005/Atom">
<
title>Example Feed</title>
<link href="http://example.org/"/>
<updated>2003-12-13T18:30:02Z</updated>
<author>
<name>John Doe</name>
<author>
</id>urn:uuid:60a76c80-d399-11d9-b93C-0003939e0af6</id>
<
entry>
<title>Atom-Powered Robots Run Amok</title>
<link href="http://example.org/2003/12/13/atom03"/>
<id>urn:uuid:1225c695-cfb8-4ebb-aaaa-80da344efa6a</id>
<updated>2003-12-13T18:30:02Z</updated>
<summary>Some text.</summary>
<entry>
</
feed> </
The default is to return 10 results. We can change this using the parameter max_results
.
= 'https://export.arxiv.org/api/query?search_query=all:large+language+model&max_results=1000'
xml_url = requests.get(xml_url) req
0:500] req.content[
b'<?xml version="1.0" encoding="UTF-8"?>\n<feed xmlns="http://www.w3.org/2005/Atom">\n <link href="http://arxiv.org/api/query?search_query%3Dall%3Alarge%20language%20model%26id_list%3D%26start%3D0%26max_results%3D1000" rel="self" type="application/atom+xml"/>\n <title type="html">ArXiv Query: search_query=all:large language model&id_list=&start=0&max_results=1000</title>\n <id>http://arxiv.org/api/61VIJItbdgya5OJr9pBClmPV2AU</id>\n <updated>2025-04-24T00:00:00-04:00</updated>\n <opensea'
Let’s “pretty print” this xml file.
from lxml import etree
# Parse the XML content
= etree.XMLParser(remove_blank_text=True)
parser = etree.fromstring(req.content, parser)
root
# Pretty print everything (commented out for website display)
# print(etree.tostring(root, pretty_print=True, encoding='utf-8').decode('utf-8'))
# Pretty print the first 9 children
for i in range(8):
= root[i]
child print(etree.tostring(child, pretty_print=True, encoding='utf-8').decode('utf-8'))
<link xmlns="http://www.w3.org/2005/Atom" href="http://arxiv.org/api/query?search_query%3Dall%3Alarge%20language%20model%26id_list%3D%26start%3D0%26max_results%3D1000" rel="self" type="application/atom+xml"/>
<title xmlns="http://www.w3.org/2005/Atom" type="html">ArXiv Query: search_query=all:large language model&id_list=&start=0&max_results=1000</title>
<id xmlns="http://www.w3.org/2005/Atom">http://arxiv.org/api/61VIJItbdgya5OJr9pBClmPV2AU</id>
<updated xmlns="http://www.w3.org/2005/Atom">2025-04-24T00:00:00-04:00</updated>
<opensearch:totalResults xmlns:opensearch="http://a9.com/-/spec/opensearch/1.1/" xmlns="http://www.w3.org/2005/Atom">1255177</opensearch:totalResults>
<opensearch:startIndex xmlns:opensearch="http://a9.com/-/spec/opensearch/1.1/" xmlns="http://www.w3.org/2005/Atom">0</opensearch:startIndex>
<opensearch:itemsPerPage xmlns:opensearch="http://a9.com/-/spec/opensearch/1.1/" xmlns="http://www.w3.org/2005/Atom">1000</opensearch:itemsPerPage>
<entry xmlns="http://www.w3.org/2005/Atom">
<id>http://arxiv.org/abs/2306.07377v1</id>
<updated>2023-06-12T19:10:47Z</updated>
<published>2023-06-12T19:10:47Z</published>
<title>Lost in Translation: Large Language Models in Non-English Content
Analysis</title>
<summary> In recent years, large language models (e.g., Open AI's GPT-4, Meta's LLaMa,
Google's PaLM) have become the dominant approach for building AI systems to
analyze and generate language online. However, the automated systems that
increasingly mediate our interactions online -- such as chatbots, content
moderation systems, and search engines -- are primarily designed for and work
far more effectively in English than in the world's other 7,000 languages.
Recently, researchers and technology companies have attempted to extend the
capabilities of large language models into languages other than English by
building what are called multilingual language models.
In this paper, we explain how these multilingual language models work and
explore their capabilities and limits. Part I provides a simple technical
explanation of how large language models work, why there is a gap in available
data between English and other languages, and how multilingual language models
attempt to bridge that gap. Part II accounts for the challenges of doing
content analysis with large language models in general and multilingual
language models in particular. Part III offers recommendations for companies,
researchers, and policymakers to keep in mind when considering researching,
developing and deploying large and multilingual language models.
</summary>
<author>
<name>Gabriel Nicholas</name>
</author>
<author>
<name>Aliya Bhatia</name>
</author>
<arxiv:comment xmlns:arxiv="http://arxiv.org/schemas/atom">50 pages, 4 figures</arxiv:comment>
<link href="http://arxiv.org/abs/2306.07377v1" rel="alternate" type="text/html"/>
<link title="pdf" href="http://arxiv.org/pdf/2306.07377v1" rel="related" type="application/pdf"/>
<arxiv:primary_category xmlns:arxiv="http://arxiv.org/schemas/atom" term="cs.CL" scheme="http://arxiv.org/schemas/atom"/>
<category term="cs.CL" scheme="http://arxiv.org/schemas/atom"/>
<category term="cs.AI" scheme="http://arxiv.org/schemas/atom"/>
</entry>
Another option to move forward is to use the xmltodict
package that translates an XML string into a Python dictionary for futher processing.
First, install in your conda environment:
pip install xmltodict
In Python:
import xmltodict
= xmltodict.parse(req.content)
dictionary dictionary.keys()
dict_keys(['feed'])
'feed'].keys() dictionary[
dict_keys(['@xmlns', 'link', 'title', 'id', 'updated', 'opensearch:totalResults', 'opensearch:startIndex', 'opensearch:itemsPerPage', 'entry'])
'feed']['entry'][0] dictionary[
{'id': 'http://arxiv.org/abs/2306.07377v1',
'updated': '2023-06-12T19:10:47Z',
'published': '2023-06-12T19:10:47Z',
'title': 'Lost in Translation: Large Language Models in Non-English Content\n Analysis',
'summary': "In recent years, large language models (e.g., Open AI's GPT-4, Meta's LLaMa,\nGoogle's PaLM) have become the dominant approach for building AI systems to\nanalyze and generate language online. However, the automated systems that\nincreasingly mediate our interactions online -- such as chatbots, content\nmoderation systems, and search engines -- are primarily designed for and work\nfar more effectively in English than in the world's other 7,000 languages.\nRecently, researchers and technology companies have attempted to extend the\ncapabilities of large language models into languages other than English by\nbuilding what are called multilingual language models.\n In this paper, we explain how these multilingual language models work and\nexplore their capabilities and limits. Part I provides a simple technical\nexplanation of how large language models work, why there is a gap in available\ndata between English and other languages, and how multilingual language models\nattempt to bridge that gap. Part II accounts for the challenges of doing\ncontent analysis with large language models in general and multilingual\nlanguage models in particular. Part III offers recommendations for companies,\nresearchers, and policymakers to keep in mind when considering researching,\ndeveloping and deploying large and multilingual language models.",
'author': [{'name': 'Gabriel Nicholas'}, {'name': 'Aliya Bhatia'}],
'arxiv:comment': {'@xmlns:arxiv': 'http://arxiv.org/schemas/atom',
'#text': '50 pages, 4 figures'},
'link': [{'@href': 'http://arxiv.org/abs/2306.07377v1',
'@rel': 'alternate',
'@type': 'text/html'},
{'@title': 'pdf',
'@href': 'http://arxiv.org/pdf/2306.07377v1',
'@rel': 'related',
'@type': 'application/pdf'}],
'arxiv:primary_category': {'@xmlns:arxiv': 'http://arxiv.org/schemas/atom',
'@term': 'cs.CL',
'@scheme': 'http://arxiv.org/schemas/atom'},
'category': [{'@term': 'cs.CL', '@scheme': 'http://arxiv.org/schemas/atom'},
{'@term': 'cs.AI', '@scheme': 'http://arxiv.org/schemas/atom'}]}
pd.DataFrame(['url': entry['id'], 'title': entry['title'], 'date': entry['published']}
{for entry in dictionary['feed']['entry']
]).head()
url | title | date | |
---|---|---|---|
0 | http://arxiv.org/abs/2306.07377v1 | Lost in Translation: Large Language Models in ... | 2023-06-12T19:10:47Z |
1 | http://arxiv.org/abs/2202.03371v1 | Cedille: A large autoregressive French languag... | 2022-02-07T17:40:43Z |
2 | http://arxiv.org/abs/2305.06530v1 | How Good are Commercial Large Language Models ... | 2023-05-11T02:29:53Z |
3 | http://arxiv.org/abs/2408.10441v1 | Goldfish: Monolingual Language Models for 350 ... | 2024-08-19T22:31:21Z |
4 | http://arxiv.org/abs/2404.09579v1 | Modelling Language | 2024-04-15T08:40:01Z |
Geo Coding
API providers
Radar (among many others) provides a free API to encode an address or a location string into precise geolocation information such as latitude/longitude pairs, or vice versa.
Forward geocode
Forward geocode takes an address and returns address data such as latitude, longitude and more.
Note: the authentication level is Publishable
so the appropriate API key is “Test Publishable” API key.
with open('radar_api_tp.txt') as file:
= file.read().strip() API_KEY
= 'https://api.radar.io/v1/geocode/forward?query=20+jay+st+brooklyn+ny'
api_url = {'Authorization': API_KEY}
headers
= requests.get(api_url, headers=headers)
req req
<Response [200]>
req.content
b'{"meta":{"code":200},"addresses":[{"addressLabel":"20 Jay St","number":"20","street":"Jay St","city":"Brooklyn","state":"New York","stateCode":"NY","postalCode":"11201","county":"Kings","countryCode":"US","formattedAddress":"20 Jay St, Brooklyn, NY 11201 US","layer":"address","latitude":40.7040913980001,"longitude":-73.986693719,"geometry":{"type":"Point","coordinates":[-73.986693719,40.7040913980001]},"distance":19716.122920923655,"confidence":"exact","country":"United States","countryFlag":"\xf0\x9f\x87\xba\xf0\x9f\x87\xb8","timeZone":{"id":"America/New_York","name":"Eastern Daylight Time","code":"EDT","currentTime":"2025-04-24T16:03:15-04:00","utcOffset":-14400,"dstOffset":3600}}]}'
req.json()
{'meta': {'code': 200},
'addresses': [{'addressLabel': '20 Jay St',
'number': '20',
'street': 'Jay St',
'city': 'Brooklyn',
'state': 'New York',
'stateCode': 'NY',
'postalCode': '11201',
'county': 'Kings',
'countryCode': 'US',
'formattedAddress': '20 Jay St, Brooklyn, NY 11201 US',
'layer': 'address',
'latitude': 40.7040913980001,
'longitude': -73.986693719,
'geometry': {'type': 'Point',
'coordinates': [-73.986693719, 40.7040913980001]},
'distance': 19716.122920923655,
'confidence': 'exact',
'country': 'United States',
'countryFlag': '🇺🇸',
'timeZone': {'id': 'America/New_York',
'name': 'Eastern Daylight Time',
'code': 'EDT',
'currentTime': '2025-04-24T16:03:15-04:00',
'utcOffset': -14400,
'dstOffset': 3600}}]}
'addresses'][0]['latitude'] req.json()[
40.7040913980001
'addresses'][0]['longitude'] req.json()[
-73.986693719
Reverse geocode
Reverse geocode takes a latitude and longitude and returns an address.
= 'https://api.radar.io/v1/geocode/reverse?coordinates=40.70390,-73.98670'
api_url = {'Authorization': API_KEY}
headers
= requests.get(api_url, headers=headers)
req req
<Response [200]>
req.json()
{'meta': {'code': 200},
'addresses': [{'addressLabel': '26 Jay St',
'number': '26',
'street': 'Jay St',
'city': 'Brooklyn',
'state': 'New York',
'stateCode': 'NY',
'postalCode': '11201',
'county': 'Kings',
'countryCode': 'US',
'formattedAddress': '26 Jay St, Brooklyn, NY 11201 US',
'layer': 'address',
'latitude': 40.704045045,
'longitude': -73.986709935,
'geometry': {'type': 'Point', 'coordinates': [-73.986709935, 40.704045045]},
'distance': 16.15506679691174,
'country': 'United States',
'countryFlag': '🇺🇸',
'timeZone': {'id': 'America/New_York',
'name': 'Eastern Daylight Time',
'code': 'EDT',
'currentTime': '2025-04-24T16:03:15-04:00',
'utcOffset': -14400,
'dstOffset': 3600}}]}
geopy
package
geopy
is a Python package (website) that provides a unified access to many geocoding API providers, as well as a wrapper to geocode addresses without explicitly managing HTTP requests.
Install in your conda environment:
pip install geopy
Most geocoding API providers require an account and/or an API key to associate the API calls to a particular account. Nominatim is a provider that provides a few API calls for free.
from geopy.geocoders import Nominatim
= Nominatim(user_agent="professor_data_science_project")
geolocator = geolocator.geocode("175 5th Avenue NYC")
location location
Location(Flatiron Building, 175, 5th Avenue, Flatiron District, Manhattan Community Board 5, Manhattan, New York County, City of New York, New York, 10010, United States, (40.7410592, -73.9896416, 0.0))
import seaborn as sns
= sns.load_dataset('penguins')
penguins penguins.island.unique()
array(['Torgersen', 'Biscoe', 'Dream'], dtype=object)
=[
geo_information + ' island')
geolocator.geocode(island for island in penguins.island.unique()
] geo_information
[Location(Torgersen Island, (-64.7727428, -64.0746078, 0.0)),
Location(Biscoe Islands, (-65.8925785, -66.440456, 0.0)),
Location(Dream Island, Hunterston, Shire of Wellington, Victoria, 3971, Australia, (-38.658886, 146.8513696, 0.0))]
0] geo_information[
Location(Torgersen Island, (-64.7727428, -64.0746078, 0.0))
0].latitude geo_information[
-64.7727428
0].longitude geo_information[
-64.0746078
0] geo_information[
Location(Torgersen Island, (-64.7727428, -64.0746078, 0.0))
= []
island_list for island in geo_information:
= island.address.split(',')[0]
address dict(address=address,
island_list.append(=island.latitude,
latitude=island.longitude)) longitude
= pd.DataFrame(island_list) island_df
='latitude', lon='longitude') px.scatter_geo(island_df, lat
Resources
Here are some other potentially useful APIs: